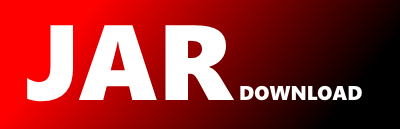
javaxt.webservices.Parameter Maven / Gradle / Ivy
package javaxt.webservices;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import javaxt.utils.Value;
import javaxt.xml.DOM;
//******************************************************************************
//** Parameter Class
//******************************************************************************
/**
* Used to represent a parameter associated with a web method.
*
******************************************************************************/
public class Parameter {
private String name;
private String type;
private Object value;
private int minOccurs = 0;
private int maxOccurs = 1;
protected boolean IsNillable;
protected boolean IsAttribute;
private Parameter[] children = null;
private Option[] options = null;
private Node parentNode;
// **************************************************************************
// ** Constructor
// **************************************************************************
/**
* Instantiates this class using a "Parameter" node from an SSD.
*/
protected Parameter(Node ParameterNode) throws InstantiationException {
if (!ParameterNode.getNodeName().equalsIgnoreCase("parameter")) {
throw new InstantiationException(DOM.getText(ParameterNode));
}
this.parentNode = ParameterNode.getParentNode();
NamedNodeMap attr = ParameterNode.getAttributes();
name = DOM.getAttributeValue(attr, "name");
type = DOM.getAttributeValue(attr, "type");
IsAttribute = bool(DOM.getAttributeValue(attr, "isattribute"));
IsNillable = bool(DOM.getAttributeValue(attr, "isnillable"));
if (name.length() == 0) {
throw new InstantiationException(DOM.getText(ParameterNode));
}
// Get min occurances
try {
minOccurs = cint(DOM.getAttributeValue(attr, "minOccurs").trim());
if (minOccurs < 0)
minOccurs = 0;
} catch (Exception e) {
}
// Get max occurances
String maxOccurs = DOM.getAttributeValue(attr, "maxOccurs").trim();
if (maxOccurs.equalsIgnoreCase("unbounded")) {
this.maxOccurs = 32767;
} else if (maxOccurs.equals("")) {
this.maxOccurs = 1;
} else {
try {
this.maxOccurs = cint(maxOccurs);
if (this.maxOccurs < 0)
this.maxOccurs = 0;
} catch (Exception e) {
}
}
// Get children
java.util.ArrayList params = new java.util.ArrayList();
for (Node node : DOM.getNodes(ParameterNode.getChildNodes())) {
if (node.getNodeName().equalsIgnoreCase("parameter")) {
try {
params.add(new Parameter(node));
} catch (InstantiationException e) {
e.printStackTrace();
}
}
// params.add(new Parameter(node));
}
if (!params.isEmpty()) {
children = params.toArray(new Parameter[params.size()]);
}
// Get list of possible values (i.e. options)
java.util.ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy