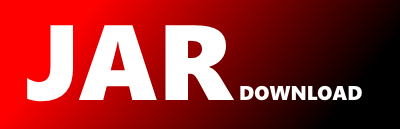
META-INF.modules.java.base.classes.java.lang.ref.Reference Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java.base Show documentation
Show all versions of java.base Show documentation
Bytecoder java.base Module
/*
* Copyright (c) 1997, 2018, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores, CA 94065 USA
* or visit www.oracle.com if you need additional information or have any
* questions.
*/
package java.lang.ref;
import jdk.internal.vm.annotation.ForceInline;
import jdk.internal.HotSpotIntrinsicCandidate;
import jdk.internal.access.JavaLangRefAccess;
import jdk.internal.access.SharedSecrets;
import jdk.internal.ref.Cleaner;
/**
* Abstract base class for reference objects. This class defines the
* operations common to all reference objects. Because reference objects are
* implemented in close cooperation with the garbage collector, this class may
* not be subclassed directly.
*
* @author Mark Reinhold
* @since 1.2
*/
public abstract class Reference {
/* The state of a Reference object is characterized by two attributes. It
* may be either "active", "pending", or "inactive". It may also be
* either "registered", "enqueued", "dequeued", or "unregistered".
*
* Active: Subject to special treatment by the garbage collector. Some
* time after the collector detects that the reachability of the
* referent has changed to the appropriate state, the collector
* "notifies" the reference, changing the state to either "pending" or
* "inactive".
* referent != null; discovered = null, or in GC discovered list.
*
* Pending: An element of the pending-Reference list, waiting to be
* processed by the ReferenceHandler thread. The pending-Reference
* list is linked through the discovered fields of references in the
* list.
* referent = null; discovered = next element in pending-Reference list.
*
* Inactive: Neither Active nor Pending.
* referent = null.
*
* Registered: Associated with a queue when created, and not yet added
* to the queue.
* queue = the associated queue.
*
* Enqueued: Added to the associated queue, and not yet removed.
* queue = ReferenceQueue.ENQUEUE; next = next entry in list, or this to
* indicate end of list.
*
* Dequeued: Added to the associated queue and then removed.
* queue = ReferenceQueue.NULL; next = this.
*
* Unregistered: Not associated with a queue when created.
* queue = ReferenceQueue.NULL.
*
* The collector only needs to examine the referent field and the
* discovered field to determine whether a (non-FinalReference) Reference
* object needs special treatment. If the referent is non-null and not
* known to be live, then it may need to be discovered for possible later
* notification. But if the discovered field is non-null, then it has
* already been discovered.
*
* FinalReference (which exists to support finalization) differs from
* other references, because a FinalReference is not cleared when
* notified. The referent being null or not cannot be used to distinguish
* between the active state and pending or inactive states. However,
* FinalReferences do not support enqueue(). Instead, the next field of a
* FinalReference object is set to "this" when it is added to the
* pending-Reference list. The use of "this" as the value of next in the
* enqueued and dequeued states maintains the non-active state. An
* additional check that the next field is null is required to determine
* that a FinalReference object is active.
*
* Initial states:
* [active/registered]
* [active/unregistered] [1]
*
* Transitions:
* clear
* [active/registered] -------> [inactive/registered]
* | |
* | | enqueue [2]
* | GC enqueue [2] |
* | -----------------|
* | |
* v |
* [pending/registered] --- v
* | | ReferenceHandler
* | enqueue [2] |---> [inactive/enqueued]
* v | |
* [pending/enqueued] --- |
* | | poll/remove
* | poll/remove |
* | |
* v ReferenceHandler v
* [pending/dequeued] ------> [inactive/dequeued]
*
*
* clear/enqueue/GC [3]
* [active/unregistered] ------
* | |
* | GC |
* | |--> [inactive/unregistered]
* v |
* [pending/unregistered] ------
* ReferenceHandler
*
* Terminal states:
* [inactive/dequeued]
* [inactive/unregistered]
*
* Unreachable states (because enqueue also clears):
* [active/enqeued]
* [active/dequeued]
*
* [1] Unregistered is not permitted for FinalReferences.
*
* [2] These transitions are not possible for FinalReferences, making
* [pending/enqueued] and [pending/dequeued] unreachable, and
* [inactive/registered] terminal.
*
* [3] The garbage collector may directly transition a Reference
* from [active/unregistered] to [inactive/unregistered],
* bypassing the pending-Reference list.
*/
private T referent; /* Treated specially by GC */
/* The queue this reference gets enqueued to by GC notification or by
* calling enqueue().
*
* When registered: the queue with which this reference is registered.
* enqueued: ReferenceQueue.ENQUEUE
* dequeued: ReferenceQueue.NULL
* unregistered: ReferenceQueue.NULL
*/
volatile ReferenceQueue super T> queue;
/* The link in a ReferenceQueue's list of Reference objects.
*
* When registered: null
* enqueued: next element in queue (or this if last)
* dequeued: this (marking FinalReferences as inactive)
* unregistered: null
*/
@SuppressWarnings("rawtypes")
volatile Reference next;
/* Used by the garbage collector to accumulate Reference objects that need
* to be revisited in order to decide whether they should be notified.
* Also used as the link in the pending-Reference list. The discovered
* field and the next field are distinct to allow the enqueue() method to
* be applied to a Reference object while it is either in the
* pending-Reference list or in the garbage collector's discovered set.
*
* When active: null or next element in a discovered reference list
* maintained by the GC (or this if last)
* pending: next element in the pending-Reference list (null if last)
* inactive: null
*/
private transient Reference discovered;
/* High-priority thread to enqueue pending References
*/
private static class ReferenceHandler extends Thread {
private static void ensureClassInitialized(Class> clazz) {
try {
Class.forName(clazz.getName(), true, clazz.getClassLoader());
} catch (ClassNotFoundException e) {
throw (Error) new NoClassDefFoundError(e.getMessage()).initCause(e);
}
}
static {
// pre-load and initialize Cleaner class so that we don't
// get into trouble later in the run loop if there's
// memory shortage while loading/initializing it lazily.
ensureClassInitialized(Cleaner.class);
}
ReferenceHandler(ThreadGroup g, String name) {
super(g, null, name, 0, false);
}
public void run() {
while (true) {
processPendingReferences();
}
}
}
/*
* Atomically get and clear (set to null) the VM's pending-Reference list.
*/
private static native Reference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy