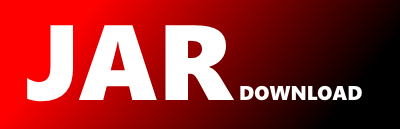
tinylaf-1_4_0_src.src.de.muntjak.tinylookandfeel.ThemeDescription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tinylaf Show documentation
Show all versions of tinylaf Show documentation
A (mostly painted) Look and Feel for Java 1.4 and higher.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy