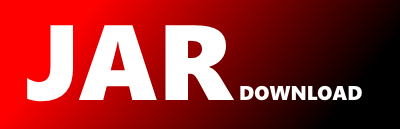
de.odysseus.staxon.json.JsonXMLConfig Maven / Gradle / Ivy
/*
* Copyright 2011 Odysseus Software GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.odysseus.staxon.json;
import javax.xml.namespace.QName;
/**
* Json XML factory configuration interface.
* A JsonXMLConfig
instance can be used to configure either
* of JsonXMLInputFactory
or JsonXMLOutputFactory
.
*
* Default values are defined by static {@link #DEFAULT} instance.
* @see JsonXMLInputFactory
* @see JsonXMLOutputFactory
*/
public interface JsonXMLConfig {
/**
*
Default configuration:
*
* - autoArray -
false
* - multiplePI -
true
* - namespaceDeclarations -
true
* - namespaceSeparator -
':'
* - prettyPrint -
false
* - virtualRoot -
null
*
*/
public static final JsonXMLConfig DEFAULT = new JsonXMLConfig() {
@Override
public boolean isAutoArray() {
return false;
}
@Override
public boolean isMultiplePI() {
return true;
}
@Override
public boolean isNamespaceDeclarations() {
return true;
}
@Override
public char getNamespaceSeparator() {
return ':';
}
@Override
public boolean isPrettyPrint() {
return false;
}
@Override
public QName getVirtualRoot() {
return null;
}
};
/**
* Trigger arrays automatically?
* @see JsonXMLOutputFactory#PROP_AUTO_ARRAY
* @return auto array flag
*/
public boolean isAutoArray();
/**
* Whether to use the {@link JsonXMLStreamConstants#MULTIPLE_PI_TARGET}
* processing instruction to indicate an array start.
* If true
, a PI is used to inform the writer to begin an array,
* passing the name of following multiple elements as data.
* The writer will close arrays automatically.
* If true
, this reader will insert a PI with the field
* name as PI data.
*
* Note that the element given in the PI may occur zero times,
* indicating an "empty array".
* @see JsonXMLInputFactory#PROP_MULTIPLE_PI
* @see JsonXMLOutputFactory#PROP_MULTIPLE_PI
* @return multiple PI flag
*/
public boolean isMultiplePI();
/**
* Whether to write namespace declarations.
* @see JsonXMLOutputFactory#PROP_NAMESPACE_DECLARATIONS
* @return namespace declarations flag
*/
public boolean isNamespaceDeclarations();
/**
* Namespace prefix separator.
* @see JsonXMLInputFactory#PROP_NAMESPACE_SEPARATOR
* @see JsonXMLOutputFactory#PROP_NAMESPACE_SEPARATOR
* @return namespace separator
*/
public char getNamespaceSeparator();
/**
* Format output for better readability?
* @see JsonXMLOutputFactory#PROP_PRETTY_PRINT
* @return pretty print flag
*/
public boolean isPrettyPrint();
/**
* JSON documents may have have multiple root properties. However,
* XML requires a single root element. This property specifies
* the root as a "virtual" element, which will be removed from the stream
* when writing and added to the stream when reading.
* @see JsonXMLInputFactory#PROP_VIRTUAL_ROOT
* @see JsonXMLOutputFactory#PROP_VIRTUAL_ROOT
* @return virtual root
*/
public QName getVirtualRoot();
}