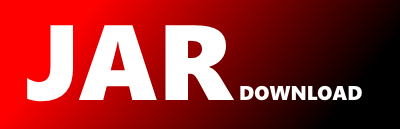
de.pitkley.jmccs.monitor.MonitorHelper Maven / Gradle / Ivy
package de.pitkley.jmccs.monitor;
import java.io.IOException;
import java.lang.IllegalStateException;
import java.lang.Override;
import java.lang.SuppressWarnings;
@SuppressWarnings("unused")
public class MonitorHelper implements Monitor {
private final Monitor monitor;
public MonitorHelper(Monitor monitor) {
this.monitor = monitor;
}
@Override
public boolean isMainMonitor() {
return monitor.isMainMonitor();
}
@Override
public boolean isVCPCodeSupported(VCPCode vcpCode) {
return monitor.isVCPCodeSupported(vcpCode);
}
@Override
public VCPReply getVCPFeature(VCPCode vcpCode) {
return monitor.getVCPFeature(vcpCode);
}
@Override
public boolean setVCPFeature(VCPCode vcpCode, int value) {
return monitor.setVCPFeature(vcpCode, value);
}
@Override
public boolean isClosed() {
return monitor.isClosed();
}
@Override
public void close() throws IOException {
monitor.close();
}
/**
* Restore all factory presets including luminance / contrast, geometry, color and TV defaults.
*
* Any non-zero value causes defaults to be restored. A value of zero must be ignored
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setRestoreFactoryDefaults(int value) {
VCPCode c = VCPCode.RESTORE_FACTORY_DEFAULTS;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Restore factory defaults for luminance and contrast adjustments.
*
* Any non-zero value causes defaults to be restored. A value of zero must be ignored
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setRestoreFactoryLuminanceContrastDefaults(int value) {
VCPCode c = VCPCode.RESTORE_FACTORY_LUMINANCE_CONTRAST_DEFAULTS;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Restore factory defaults for geometry adjustments.
*
* Any non-zero value causes defaults to be restored. A value of zero must be ignored
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setRestoreFactoryGeometryDefaults(int value) {
VCPCode c = VCPCode.RESTORE_FACTORY_GEOMETRY_DEFAULTS;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Restore factory defaults for color settings.
*
* Any non-zero value causes defaults to be restored. A value of zero must be ignored
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setRestoreFactoryColorDefaults(int value) {
VCPCode c = VCPCode.RESTORE_FACTORY_COLOR_DEFAULTS;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Restore factory defaults for TV functions.
*
* Any non-zero value causes defaults to be restored. A value of zero must be ignored
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setRestoreFactoryTvDefaults(int value) {
VCPCode c = VCPCode.RESTORE_FACTORY_TV_DEFAULTS;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Store / Restore the user saved values for current mode.
*
*
* Byte: SL Description
*
* 01h Store current settings in the monitor.
* 02h Restore factory defaults for current mode. If no factory defaults then restore user values for current mode.
*
*
* All other values are reserved and must be ignored.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSettings(int value) {
VCPCode c = VCPCode.SETTINGS;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Allows the display to specify the minimum increment in which it can adjust the color temperature.
*
* This will be used in conjunction with VCP code 0Ch, Color temperature request.
*
* Values of 0 and > 5000 are invalid and must be ignored.
*
* @return the reply to the sent command
*/
public VCPReply getColorTemperatureIncrement() {
return monitor.getVCPFeature(VCPCode.COLOR_TEMPERATURE_INCREMENT);
}
/**
* Allows a specified color temperature (in K) to be requested. If display is unable to achieve requested color temperature, then it should move to the closest possible temperature.
*
* A value of 0 must be treated as a request for a color temperature of 3000 K. Values greater than 0 must be used as a multiplier of the color temperature increment (read using VCP 0Bh) and the result added to the base value of 3000 K
*
* Example:
*
* If VCP 0Bh returns a value of 50 K and VCP code 0Ch sends a value of 50 (decimal) then the display must interpret this as a request to adjust the color temperature to 5500 K
*
* (3000 + (50 * 50)) K = 5500 K
*
* Notes:
*
*
* - Applications using this function are recommended to read the actual color temperature after using this command and taking appropriate action.
* - This control is only recommended if the display can produce a continuously (at defined increment, see VCP code 0Bh) variable color temperature.
*
*
* @return the reply to the sent command
*/
public VCPReply getColorTemperatureRequest() {
return monitor.getVCPFeature(VCPCode.COLOR_TEMPERATURE_REQUEST);
}
/**
* Allows a specified color temperature (in K) to be requested. If display is unable to achieve requested color temperature, then it should move to the closest possible temperature.
*
* A value of 0 must be treated as a request for a color temperature of 3000 K. Values greater than 0 must be used as a multiplier of the color temperature increment (read using VCP 0Bh) and the result added to the base value of 3000 K
*
* Example:
*
* If VCP 0Bh returns a value of 50 K and VCP code 0Ch sends a value of 50 (decimal) then the display must interpret this as a request to adjust the color temperature to 5500 K
*
* (3000 + (50 * 50)) K = 5500 K
*
* Notes:
*
*
* - Applications using this function are recommended to read the actual color temperature after using this command and taking appropriate action.
* - This control is only recommended if the display can produce a continuously (at defined increment, see VCP code 0Bh) variable color temperature.
*
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setColorTemperatureRequest(int value) {
VCPCode c = VCPCode.COLOR_TEMPERATURE_REQUEST;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the video sampling clock frequency
*
* @return the reply to the sent command
*/
public VCPReply getClock() {
return monitor.getVCPFeature(VCPCode.CLOCK);
}
/**
* Increasing (decreasing) this value will increase (decrease) the video sampling clock frequency
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setClock(int value) {
VCPCode c = VCPCode.CLOCK;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the Luminance of the image.
*
* @return the reply to the sent command
*/
public VCPReply getLuminance() {
return monitor.getVCPFeature(VCPCode.LUMINANCE);
}
/**
* Increasing (decreasing) this value will increase (decrease) the Luminance of the image.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setLuminance(int value) {
VCPCode c = VCPCode.LUMINANCE;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the Contrast of the image.
*
* Notes:
*
*
* - The actual range of contrast over which this control applies is defined by the manufacturer.
* - Care should be taken to avoid the situation where the contrast ratio approaches 0 … this may be non-recoverable since user will not be able to see the image.
*
*
* @return the reply to the sent command
*/
public VCPReply getContrast() {
return monitor.getVCPFeature(VCPCode.CONTRAST);
}
/**
* Increasing (decreasing) this value will increase (decrease) the Contrast of the image.
*
* Notes:
*
*
* - The actual range of contrast over which this control applies is defined by the manufacturer.
* - Care should be taken to avoid the situation where the contrast ratio approaches 0 … this may be non-recoverable since user will not be able to see the image.
*
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setContrast(int value) {
VCPCode c = VCPCode.CONTRAST;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the luminance of red pixels.
*
* The value returned must be an indication of the actual red gain at the current color temperature and not be normalized.
*
* @return the reply to the sent command
*/
public VCPReply getVideoGainDriveRed() {
return monitor.getVCPFeature(VCPCode.VIDEO_GAIN_DRIVE_RED);
}
/**
* Increasing (decreasing) this value will increase (decrease) the luminance of red pixels.
*
* The value returned must be an indication of the actual red gain at the current color temperature and not be normalized.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setVideoGainDriveRed(int value) {
VCPCode c = VCPCode.VIDEO_GAIN_DRIVE_RED;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the degree of compensation.
*
* Note:
*
* This is intended to help user suffering from the form of color deficiency in which red colors are poorly seen.
*
* @return the reply to the sent command
*/
public VCPReply getUserColorVisionCompensation() {
return monitor.getVCPFeature(VCPCode.USER_COLOR_VISION_COMPENSATION);
}
/**
* Increasing (decreasing) this value will increase (decrease) the degree of compensation.
*
* Note:
*
* This is intended to help user suffering from the form of color deficiency in which red colors are poorly seen.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setUserColorVisionCompensation(int value) {
VCPCode c = VCPCode.USER_COLOR_VISION_COMPENSATION;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the luminance of green pixels.
*
* The value returned must be an indication of the actual green gain at the current color temperature and not be normalized.
*
* @return the reply to the sent command
*/
public VCPReply getVideoGainDriveGreen() {
return monitor.getVCPFeature(VCPCode.VIDEO_GAIN_DRIVE_GREEN);
}
/**
* Increasing (decreasing) this value will increase (decrease) the luminance of green pixels.
*
* The value returned must be an indication of the actual green gain at the current color temperature and not be normalized.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setVideoGainDriveGreen(int value) {
VCPCode c = VCPCode.VIDEO_GAIN_DRIVE_GREEN;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the luminance of blue pixels.
*
* The value returned must be an indication of the actual blue gain at the current color temperature and not be normalized.
*
* @return the reply to the sent command
*/
public VCPReply getVideoGainDriveBlue() {
return monitor.getVCPFeature(VCPCode.VIDEO_GAIN_DRIVE_BLUE);
}
/**
* Increasing (decreasing) this value will increase (decrease) the luminance of blue pixels.
*
* The value returned must be an indication of the actual blue gain at the current color temperature and not be normalized.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setVideoGainDriveBlue(int value) {
VCPCode c = VCPCode.VIDEO_GAIN_DRIVE_BLUE;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will adjust the focus of the image.
*
* @return the reply to the sent command
*/
public VCPReply getFocus() {
return monitor.getVCPFeature(VCPCode.FOCUS);
}
/**
* Increasing (decreasing) this value will adjust the focus of the image.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setFocus(int value) {
VCPCode c = VCPCode.FOCUS;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Perform auto setup function (H/V position, clock, clock phase, A/D converter, etc)
*
*
* Byte: SL Description
*
* 00h Auto setup is not active
* 01h Perform / performing auto setup
* 02h Enable continuous / periodic auto setup
* >= 03h Reserved, must be ignored
*
*
* Note:
*
* A value of 02h (when supported) must cause the display to either continuously or periodically (event or timer driven) perform an auto setup. Cancel by writing a value of either 01h or 00h.
*
* @return the reply to the sent command
*/
public VCPReply getAutoSetup() {
return monitor.getVCPFeature(VCPCode.AUTO_SETUP);
}
/**
* Perform auto setup function (H/V position, clock, clock phase, A/D converter, etc)
*
*
* Byte: SL Description
*
* 00h Auto setup is not active
* 01h Perform / performing auto setup
* 02h Enable continuous / periodic auto setup
* >= 03h Reserved, must be ignored
*
*
* Note:
*
* A value of 02h (when supported) must cause the display to either continuously or periodically (event or timer driven) perform an auto setup. Cancel by writing a value of either 01h or 00h.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setAutoSetup(int value) {
VCPCode c = VCPCode.AUTO_SETUP;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Perform auto color setup function (R / G / B gain and offset, A/D setup, etc.)
*
*
* Byte: SL Description
*
* 00h Auto color setup is not active
* 01h Perform / performing auto color setup
* 02h Enable continuous / periodic auto color setup
* >= 03h Reserved, must be ignored
*
*
* Note:
*
* A value of 02h (when supported) must cause the display to either continuously or periodically (event or timer driven) perform an auto color setup. Cancel by writing a value of either 01h or 00h.
*
* @return the reply to the sent command
*/
public VCPReply getAutoColorSetup() {
return monitor.getVCPFeature(VCPCode.AUTO_COLOR_SETUP);
}
/**
* Perform auto color setup function (R / G / B gain and offset, A/D setup, etc.)
*
*
* Byte: SL Description
*
* 00h Auto color setup is not active
* 01h Perform / performing auto color setup
* 02h Enable continuous / periodic auto color setup
* >= 03h Reserved, must be ignored
*
*
* Note:
*
* A value of 02h (when supported) must cause the display to either continuously or periodically (event or timer driven) perform an auto color setup. Cancel by writing a value of either 01h or 00h.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setAutoColorSetup(int value) {
VCPCode c = VCPCode.AUTO_COLOR_SETUP;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the phase shift of the sampling clock.
*
* @return the reply to the sent command
*/
public VCPReply getClockPhase() {
return monitor.getVCPFeature(VCPCode.CLOCK_PHASE);
}
/**
* Increasing (decreasing) this value will increase (decrease) the phase shift of the sampling clock.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setClockPhase(int value) {
VCPCode c = VCPCode.CLOCK_PHASE;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value controls the horizontal picture moiré cancellation.
*
* @return the reply to the sent command
*/
public VCPReply getHorizontalMoire() {
return monitor.getVCPFeature(VCPCode.HORIZONTAL_MOIRE);
}
/**
* Increasing (decreasing) this value controls the horizontal picture moiré cancellation.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setHorizontalMoire(int value) {
VCPCode c = VCPCode.HORIZONTAL_MOIRE;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value controls the vertical picture moiré cancellation.
*
* @return the reply to the sent command
*/
public VCPReply getVerticalMoire() {
return monitor.getVCPFeature(VCPCode.VERTICAL_MOIRE);
}
/**
* Increasing (decreasing) this value controls the vertical picture moiré cancellation.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setVerticalMoire(int value) {
VCPCode c = VCPCode.VERTICAL_MOIRE;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the red saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in red saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in red saturation
*
*
* If set = 7fh then display must make no change to the red saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for red saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisSaturationControlRed() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_SATURATION_CONTROL_RED);
}
/**
* Adjust the red saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in red saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in red saturation
*
*
* If set = 7fh then display must make no change to the red saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for red saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisSaturationControlRed(int value) {
VCPCode c = VCPCode.SIX_AXIS_SATURATION_CONTROL_RED;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the yellow saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in yellow saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in yellow saturation
*
*
* If set = 7fh then display must make no change to the yellow saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for yellow saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisSaturationControlYellow() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_SATURATION_CONTROL_YELLOW);
}
/**
* Adjust the yellow saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in yellow saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in yellow saturation
*
*
* If set = 7fh then display must make no change to the yellow saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for yellow saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisSaturationControlYellow(int value) {
VCPCode c = VCPCode.SIX_AXIS_SATURATION_CONTROL_YELLOW;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the green saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in green saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in green saturation
*
*
* If set = 7fh then display must make no change to the green saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for green saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisSaturationControlGreen() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_SATURATION_CONTROL_GREEN);
}
/**
* Adjust the green saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in green saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in green saturation
*
*
* If set = 7fh then display must make no change to the green saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for green saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisSaturationControlGreen(int value) {
VCPCode c = VCPCode.SIX_AXIS_SATURATION_CONTROL_GREEN;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the cyan saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in cyan saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in cyan saturation
*
*
* If set = 7fh then display must make no change to the cyan saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for cyan saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisSaturationControlCyan() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_SATURATION_CONTROL_CYAN);
}
/**
* Adjust the cyan saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in cyan saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in cyan saturation
*
*
* If set = 7fh then display must make no change to the cyan saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for cyan saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisSaturationControlCyan(int value) {
VCPCode c = VCPCode.SIX_AXIS_SATURATION_CONTROL_CYAN;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the blue saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in blue saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in blue saturation
*
*
* If set = 7fh then display must make no change to the blue saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for blue saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisSaturationControlBlue() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_SATURATION_CONTROL_BLUE);
}
/**
* Adjust the blue saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in blue saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in blue saturation
*
*
* If set = 7fh then display must make no change to the blue saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for blue saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisSaturationControlBlue(int value) {
VCPCode c = VCPCode.SIX_AXIS_SATURATION_CONTROL_BLUE;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the magenta saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in magenta saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in magenta saturation
*
*
* If set = 7fh then display must make no change to the magenta saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for magenta saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisSaturationControlMagenta() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_SATURATION_CONTROL_MAGENTA);
}
/**
* Adjust the magenta saturation for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in magenta saturation
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in magenta saturation
*
*
* If set = 7fh then display must make no change to the magenta saturation of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for magenta saturation.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisSaturationControlMagenta(int value) {
VCPCode c = VCPCode.SIX_AXIS_SATURATION_CONTROL_MAGENTA;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the black level of the red video.
*
* @return the reply to the sent command
*/
public VCPReply getVideoBlackLevelRed() {
return monitor.getVCPFeature(VCPCode.VIDEO_BLACK_LEVEL_RED);
}
/**
* Increasing (decreasing) this value will increase (decrease) the black level of the red video.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setVideoBlackLevelRed(int value) {
VCPCode c = VCPCode.VIDEO_BLACK_LEVEL_RED;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the black level of the green video.
*
* @return the reply to the sent command
*/
public VCPReply getVideoBlackLevelGreen() {
return monitor.getVCPFeature(VCPCode.VIDEO_BLACK_LEVEL_GREEN);
}
/**
* Increasing (decreasing) this value will increase (decrease) the black level of the green video.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setVideoBlackLevelGreen(int value) {
VCPCode c = VCPCode.VIDEO_BLACK_LEVEL_GREEN;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the black level of the blue video.
*
* @return the reply to the sent command
*/
public VCPReply getVideoBlackLevelBlue() {
return monitor.getVCPFeature(VCPCode.VIDEO_BLACK_LEVEL_BLUE);
}
/**
* Increasing (decreasing) this value will increase (decrease) the black level of the blue video.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setVideoBlackLevelBlue(int value) {
VCPCode c = VCPCode.VIDEO_BLACK_LEVEL_BLUE;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the zoom function of the projection lens.
*
* @return the reply to the sent command
*/
public VCPReply getAdjustZoom() {
return monitor.getVCPFeature(VCPCode.ADJUST_ZOOM);
}
/**
* Increasing (decreasing) this value will increase (decrease) the zoom function of the projection lens.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setAdjustZoom(int value) {
VCPCode c = VCPCode.ADJUST_ZOOM;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Allows one of a range of algorithms to be selected to suit the type of image being displayed and/or personal preference.
*
* Increasing (decreasing) the value must increase (decrease) the edge sharpness of image features.
*
* @return the reply to the sent command
*/
public VCPReply getSharpness() {
return monitor.getVCPFeature(VCPCode.SHARPNESS);
}
/**
* Allows one of a range of algorithms to be selected to suit the type of image being displayed and/or personal preference.
*
* Increasing (decreasing) the value must increase (decrease) the edge sharpness of image features.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSharpness(int value) {
VCPCode c = VCPCode.SHARPNESS;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this value will increase (decrease) the velocity modulation of the horizontal scan as a function of a change in the luminance level.
*
* @return the reply to the sent command
*/
public VCPReply getVelocityScanModulation() {
return monitor.getVCPFeature(VCPCode.VELOCITY_SCAN_MODULATION);
}
/**
* Increasing (decreasing) this value will increase (decrease) the velocity modulation of the horizontal scan as a function of a change in the luminance level.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setVelocityScanModulation(int value) {
VCPCode c = VCPCode.VELOCITY_SCAN_MODULATION;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing this control increases the amplitude of the color difference components of the video signal.
*
* The result is an increase in the amount of pure color relative to white in the video. This control applies to the currently active interface.
*
* @return the reply to the sent command
*/
public VCPReply getColorSaturation() {
return monitor.getVCPFeature(VCPCode.COLOR_SATURATION);
}
/**
* Increasing this control increases the amplitude of the color difference components of the video signal.
*
* The result is an increase in the amount of pure color relative to white in the video. This control applies to the currently active interface.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setColorSaturation(int value) {
VCPCode c = VCPCode.COLOR_SATURATION;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing this control increases the amplitude of the high frequency components of the video signal.
*
* This allows fine details to be accentuated. This control does not affect the RGB input, only the TV video inputs.
*
* @return the reply to the sent command
*/
public VCPReply getTvSharpness() {
return monitor.getVCPFeature(VCPCode.TV_SHARPNESS);
}
/**
* Increasing this control increases the amplitude of the high frequency components of the video signal.
*
* This allows fine details to be accentuated. This control does not affect the RGB input, only the TV video inputs.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setTvSharpness(int value) {
VCPCode c = VCPCode.TV_SHARPNESS;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing (decreasing) this control increases (decreases) the ratio between whites and blacks in the video.
*
* This control does not affect the RGB input, only the TV video inputs.
*
* @return the reply to the sent command
*/
public VCPReply getTvContrast() {
return monitor.getVCPFeature(VCPCode.TV_CONTRAST);
}
/**
* Increasing (decreasing) this control increases (decreases) the ratio between whites and blacks in the video.
*
* This control does not affect the RGB input, only the TV video inputs.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setTvContrast(int value) {
VCPCode c = VCPCode.TV_CONTRAST;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Also known as tint
*
* Increasing (decreasing) this control increases (decreases) the wavelength of the color component of the video signal.
*
* The result is a shift towards red (blue) in the hue of all colors. This control applies to the currently active interface.
*
* @return the reply to the sent command
*/
public VCPReply getHue() {
return monitor.getVCPFeature(VCPCode.HUE);
}
/**
* Also known as tint
*
* Increasing (decreasing) this control increases (decreases) the wavelength of the color component of the video signal.
*
* The result is a shift towards red (blue) in the hue of all colors. This control applies to the currently active interface.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setHue(int value) {
VCPCode c = VCPCode.HUE;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Increasing this control increases the black level of the video, resulting in an increase of the luminance level of the video.
*
* A value of zero represents the darkest level possible.
*
* This control does not affect the RGB input, only the TV video inputs.
*
* @return the reply to the sent command
*/
public VCPReply getTvBlackLevelLuminance() {
return monitor.getVCPFeature(VCPCode.TV_BLACK_LEVEL_LUMINANCE);
}
/**
* Increasing this control increases the black level of the video, resulting in an increase of the luminance level of the video.
*
* A value of zero represents the darkest level possible.
*
* This control does not affect the RGB input, only the TV video inputs.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setTvBlackLevelLuminance(int value) {
VCPCode c = VCPCode.TV_BLACK_LEVEL_LUMINANCE;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Changes the contrast ratio between the area of the window and the rest of the desktop
*
* Lower (higher) values will cause the desktop luminance to decrease (increase)
*
* Notes:
*
*
* - This VCP code should be used in conjunction with VCP 99h
* - This command structure is not recommended for new designs, see VCP A5h for alternate.
*
*
* @return the reply to the sent command
*/
public VCPReply getWindowBackground() {
return monitor.getVCPFeature(VCPCode.WINDOW_BACKGROUND);
}
/**
* Changes the contrast ratio between the area of the window and the rest of the desktop
*
* Lower (higher) values will cause the desktop luminance to decrease (increase)
*
* Notes:
*
*
* - This VCP code should be used in conjunction with VCP 99h
* - This command structure is not recommended for new designs, see VCP A5h for alternate.
*
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setWindowBackground(int value) {
VCPCode c = VCPCode.WINDOW_BACKGROUND;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the red hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in red hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in red hue
*
*
* If set = 7fh then display must make no change to the red hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for red hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisHueControlRed() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_HUE_CONTROL_RED);
}
/**
* Adjust the red hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in red hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in red hue
*
*
* If set = 7fh then display must make no change to the red hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for red hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisHueControlRed(int value) {
VCPCode c = VCPCode.SIX_AXIS_HUE_CONTROL_RED;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the yellow hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in yellow hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in yellow hue
*
*
* If set = 7fh then display must make no change to the yellow hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for yellow hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisHueControlYellow() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_HUE_CONTROL_YELLOW);
}
/**
* Adjust the yellow hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in yellow hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in yellow hue
*
*
* If set = 7fh then display must make no change to the yellow hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for yellow hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisHueControlYellow(int value) {
VCPCode c = VCPCode.SIX_AXIS_HUE_CONTROL_YELLOW;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the green hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in green hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in green hue
*
*
* If set = 7fh then display must make no change to the green hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for green hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisHueControlGreen() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_HUE_CONTROL_GREEN);
}
/**
* Adjust the green hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in green hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in green hue
*
*
* If set = 7fh then display must make no change to the green hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for green hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisHueControlGreen(int value) {
VCPCode c = VCPCode.SIX_AXIS_HUE_CONTROL_GREEN;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the cyan hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in cyan hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in cyan hue
*
*
* If set = 7fh then display must make no change to the cyan hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for cyan hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisHueControlCyan() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_HUE_CONTROL_CYAN);
}
/**
* Adjust the cyan hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in cyan hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in cyan hue
*
*
* If set = 7fh then display must make no change to the cyan hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for cyan hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisHueControlCyan(int value) {
VCPCode c = VCPCode.SIX_AXIS_HUE_CONTROL_CYAN;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the blue hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in blue hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in blue hue
*
*
* If set = 7fh then display must make no change to the blue hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for blue hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisHueControlBlue() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_HUE_CONTROL_BLUE);
}
/**
* Adjust the blue hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in blue hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in blue hue
*
*
* If set = 7fh then display must make no change to the blue hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for blue hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisHueControlBlue(int value) {
VCPCode c = VCPCode.SIX_AXIS_HUE_CONTROL_BLUE;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Adjust the magenta hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in magenta hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in magenta hue
*
*
* If set = 7fh then display must make no change to the magenta hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for magenta hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @return the reply to the sent command
*/
public VCPReply getSixAxisHueControlMagenta() {
return monitor.getVCPFeature(VCPCode.SIX_AXIS_HUE_CONTROL_MAGENTA);
}
/**
* Adjust the magenta hue for 6-axis color
*
*
* Byte: SL Description
*
* > 7Fh Causes an increase in magenta hue
* 7Fh The nominal (default) value
* < 7Fh Causes a decrease in magenta hue
*
*
* If set = 7fh then display must make no change to the magenta hue of the incoming signal.
* If set != 7Fh, then writing a value = 7Fh must cause the display to return to its nominal (default) setting for magenta hue.
* The +- 7Fh range must be linearly mapped to the actual adjustment range.
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setSixAxisHueControlMagenta(int value) {
VCPCode c = VCPCode.SIX_AXIS_HUE_CONTROL_MAGENTA;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Turn on / off the auto setup function (periodic or event driven).
*
*
* Byte: SL Description
*
* 00h Reserved, must be ignored
* 01h Turn auto setup off
* 02h Turn auto setup on
* >= 03h Reserved, must be ignored
*
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setAutoSetupOnOff(int value) {
VCPCode c = VCPCode.AUTO_SETUP_ON_OFF;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
/**
* Indicates the orientation of the screen
*
*
* Byte: SL Description
*
* 00h Reserved Shall be ignored
* 01h 0 degrees The normal landscape mode
* 02h 90 degrees Portrait mode achieved by clockwise rotation of the display 90 degrees.
* 03h 180 degrees Landscape mode achieved by rotation of the display 180 degrees.
* 04h 270 degrees Portrait mode achieved by clockwise rotation of the display 270 degrees.
* 05h to FEh Reserved Shall be ignored
* FFh Not applicable Indicates that the display cannot supply the current orientation
*
*
* @return the reply to the sent command
*/
public VCPReply getScreenOrientation() {
return monitor.getVCPFeature(VCPCode.SCREEN_ORIENTATION);
}
/**
* Permits the selection of a preset optimized by manufacturer for an application type or the selection of a user defined setting.
*
*
* Byte: SL Description
*
* 00h Stand / default mode
* 01h Productivity (e.g. office applications)
* 02h Mixed (e.g. internet with mix of text and images)
* 03h Movie
* 04h User defined
* 05h Games (e.g. games console / PC game)
* 06h Sports (e.g. fast action)
* 07h Professional (all signal processing disabled)
* 08h Standard / default mode with intermediate power consumption
* 09h Standard / default mode with low power consumption
* 0Ah Demonstration (used for high visual impact in retail etc)
* 0Bh - EFh Reserved, must be ignored
* F0h Dynamic contrast
* >= F1h Reserved, must be ignored
*
*
* @return the reply to the sent command
*/
public VCPReply getDisplayApplication() {
return monitor.getVCPFeature(VCPCode.DISPLAY_APPLICATION);
}
/**
* Permits the selection of a preset optimized by manufacturer for an application type or the selection of a user defined setting.
*
*
* Byte: SL Description
*
* 00h Stand / default mode
* 01h Productivity (e.g. office applications)
* 02h Mixed (e.g. internet with mix of text and images)
* 03h Movie
* 04h User defined
* 05h Games (e.g. games console / PC game)
* 06h Sports (e.g. fast action)
* 07h Professional (all signal processing disabled)
* 08h Standard / default mode with intermediate power consumption
* 09h Standard / default mode with low power consumption
* 0Ah Demonstration (used for high visual impact in retail etc)
* 0Bh - EFh Reserved, must be ignored
* F0h Dynamic contrast
* >= F1h Reserved, must be ignored
*
*
* @param value the value to set the VCP-code to
* @return true
if the command succeeded, otherwise false
*/
public boolean setDisplayApplication(int value) {
VCPCode c = VCPCode.DISPLAY_APPLICATION;
if (!c.isValueLegal(value)) {
throw new IllegalStateException("The value '" + value + "' is illegal");
}
return monitor.setVCPFeature(c, value);
}
}