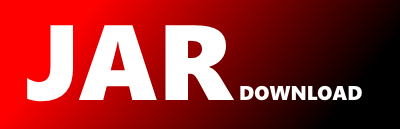
de.ppi.selenium.assertj.SeleniumSoftAssertions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of webtest Show documentation
Show all versions of webtest Show documentation
Some additional helper for webtesting with selenium
package de.ppi.selenium.assertj;
import static org.assertj.core.util.Arrays.array;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import net.sf.cglib.proxy.Enhancer;
import net.sf.cglib.proxy.MethodInterceptor;
import net.sf.cglib.proxy.MethodProxy;
import org.assertj.core.api.AbstractSoftAssertions;
import org.assertj.core.api.JUnitSoftAssertions;
import org.junit.rules.TestRule;
import org.junit.runner.Description;
import org.junit.runners.model.MultipleFailureException;
import org.junit.runners.model.Statement;
import org.openqa.selenium.Alert;
import org.selophane.elements.base.Element;
import de.ppi.selenium.browser.WebBrowser;
import de.ppi.selenium.logevent.api.EventLoggerFactory;
import de.ppi.selenium.logevent.api.EventSource;
/**
* Selenium specific assertions in the soft-variant.
*
* @see JUnitSoftAssertions
*
*/
public class SeleniumSoftAssertions extends AbstractSoftAssertions implements
TestRule {
/**
* Collector for errors.
*/
private final ErrorCollectorWithScreenshots collector =
new ErrorCollectorWithScreenshots();
/**
*
* Initiates an object of type SeleniumSoftAssertions.
*/
public SeleniumSoftAssertions() {
super();
}
/**
* Creates a new instance of {@link ElementAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public ElementAssert assertThat(Element actual) {
return proxy(ElementAssert.class, Element.class, actual);
}
/**
* Creates a new instance of {@link AlertAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public AlertAssert assertThat(Alert actual) {
return proxy(AlertAssert.class, Alert.class, actual);
}
/**
* Creates a new instance of {@link WebbrowserAssert}
.
*
* @param actual the actual value.
* @return the created assertion object.
*/
public WebbrowserAssert assertThat(WebBrowser actual) {
return proxy(WebbrowserAssert.class, WebBrowser.class, actual);
}
@Override
public Statement apply(final Statement base, Description description) {
return new Statement() {
@Override
public void evaluate() throws Throwable {
base.evaluate();
MultipleFailureException.assertEmpty(collector.errors());
}
};
}
@Override
@SuppressWarnings("unchecked")
protected V proxy(Class assertClass, Class actualClass,
T actual) {
Enhancer enhancer = new Enhancer();
enhancer.setSuperclass(assertClass);
enhancer.setCallback(collector);
return (V) enhancer.create(array(actualClass), array(actual));
}
/**
*
* Class which collects errors and create screenshots.
*
*/
static class ErrorCollectorWithScreenshots implements MethodInterceptor {
/** Eventlogger-Factory. */
private static final EventLoggerFactory EVENT_LOGGER =
EventLoggerFactory.getInstance(EventSource.ASSERTION);
/** List of errors. */
private final List errors = new ArrayList();
@Override
public Object intercept(Object obj, Method method, Object[] args,
MethodProxy proxy) throws Throwable {
try {
proxy.invokeSuper(obj, args);
} catch (AssertionError e) {
errors.add(e);
EVENT_LOGGER.onFailure(obj.getClass().getName(),
method.getName()).logAssertionError(e);
}
return obj;
}
/**
* Returns the list of errors.
*
* @return the list of errors.
*/
public List errors() {
return Collections.unmodifiableList(errors);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy