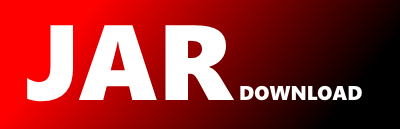
de.qytera.qtaf.cucumber.helper.CucumberTestStepHelper Maven / Gradle / Ivy
package de.qytera.qtaf.cucumber.helper;
import de.qytera.qtaf.core.reflection.FieldHelper;
import io.cucumber.plugin.event.HookTestStep;
import io.cucumber.plugin.event.PickleStepTestStep;
import io.cucumber.plugin.event.TestStep;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.UUID;
import java.util.stream.Collectors;
/**
* Helper class for extracting information from Cucumber TestStep objects.
*/
public class CucumberTestStepHelper {
private CucumberTestStepHelper() {
}
/**
* Get all test steps that are derived from the PickleStepTestStep class
* which represents Given / When / Then steps.
*
* @param testSteps List of TestStep objects
* @return List of PickleStepTestStep objects
*/
public static List getPickleStepTestSteps(List testSteps) {
return testSteps
.stream()
.filter(PickleStepTestStep.class::isInstance)
.map(ts -> (PickleStepTestStep) ts)
.toList();
}
/**
* Get the positions of test steps (Given, When, Then).
* All test steps that are defined in a feature file are represented by the PickleStepTestStep class.
* This functions finds these classes and returns their positions.
*
* @param testSteps List of test steps
* @return List of positions
*/
public static List getTestStepPositions(List testSteps) {
return testSteps
.stream()
.filter(PickleStepTestStep.class::isInstance)
.map(testSteps::indexOf)
.toList();
}
/**
* Get the positions of the given test step in a list of test steps.
*
* @param testSteps List of test steps
* @param testStep Single test steps
* @return TestStep position
*/
public static int getTestStepPosition(List testSteps, TestStep testStep) {
Optional pos = testSteps
.stream()
.filter(ts -> ts.getId().equals(testStep.getId()))
.map(testSteps::indexOf)
.findFirst();
return pos.orElse(-1);
}
/**
* Find the TestStep object that has another TestStep object with in a List stored in its 'attributeName' Attribute.
*
* @param testSteps List of TestStep objects
* @param stepId Look for this ID inside attributeName
* @param attributeName Attribute name where to look for ID
* @return TestStep object on success, null otherwise
*/
public static TestStep findByTestStepIdInAttribute(List testSteps, UUID stepId, String attributeName) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy