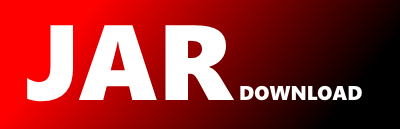
de.qytera.qtaf.testrail.entity.Attachment Maven / Gradle / Ivy
// Generated by delombok at Tue Jun 25 12:09:13 UTC 2024
package de.qytera.qtaf.testrail.entity;
import com.google.gson.annotations.SerializedName;
/**
* A TestRail attachment.
*/
public class Attachment {
private String filetype;
private String icon;
@SerializedName("entity_id")
private String entityId;
@SerializedName("client_id")
private int clientId;
@SerializedName("entity_type")
private String entityType;
private String filename;
private int size;
@SerializedName("project_id")
private int projectId;
@SerializedName("created_on")
private int createdOn;
@SerializedName("data_id")
private String dataId;
@SerializedName("user_id")
private int userId;
private String name;
@SerializedName("legacy_id")
private int legacyId;
@SerializedName("is_image")
private boolean isImage;
private String id;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFiletype() {
return this.filetype;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIcon() {
return this.icon;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEntityId() {
return this.entityId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int getClientId() {
return this.clientId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEntityType() {
return this.entityType;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFilename() {
return this.filename;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int getSize() {
return this.size;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int getProjectId() {
return this.projectId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int getCreatedOn() {
return this.createdOn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDataId() {
return this.dataId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int getUserId() {
return this.userId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int getLegacyId() {
return this.legacyId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean isImage() {
return this.isImage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFiletype(final String filetype) {
this.filetype = filetype;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIcon(final String icon) {
this.icon = icon;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEntityId(final String entityId) {
this.entityId = entityId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setClientId(final int clientId) {
this.clientId = clientId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEntityType(final String entityType) {
this.entityType = entityType;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFilename(final String filename) {
this.filename = filename;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSize(final int size) {
this.size = size;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setProjectId(final int projectId) {
this.projectId = projectId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreatedOn(final int createdOn) {
this.createdOn = createdOn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDataId(final String dataId) {
this.dataId = dataId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserId(final int userId) {
this.userId = userId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLegacyId(final int legacyId) {
this.legacyId = legacyId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setImage(final boolean isImage) {
this.isImage = isImage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Attachment)) return false;
final Attachment other = (Attachment) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (this.getClientId() != other.getClientId()) return false;
if (this.getSize() != other.getSize()) return false;
if (this.getProjectId() != other.getProjectId()) return false;
if (this.getCreatedOn() != other.getCreatedOn()) return false;
if (this.getUserId() != other.getUserId()) return false;
if (this.getLegacyId() != other.getLegacyId()) return false;
if (this.isImage() != other.isImage()) return false;
final java.lang.Object this$filetype = this.getFiletype();
final java.lang.Object other$filetype = other.getFiletype();
if (this$filetype == null ? other$filetype != null : !this$filetype.equals(other$filetype)) return false;
final java.lang.Object this$icon = this.getIcon();
final java.lang.Object other$icon = other.getIcon();
if (this$icon == null ? other$icon != null : !this$icon.equals(other$icon)) return false;
final java.lang.Object this$entityId = this.getEntityId();
final java.lang.Object other$entityId = other.getEntityId();
if (this$entityId == null ? other$entityId != null : !this$entityId.equals(other$entityId)) return false;
final java.lang.Object this$entityType = this.getEntityType();
final java.lang.Object other$entityType = other.getEntityType();
if (this$entityType == null ? other$entityType != null : !this$entityType.equals(other$entityType)) return false;
final java.lang.Object this$filename = this.getFilename();
final java.lang.Object other$filename = other.getFilename();
if (this$filename == null ? other$filename != null : !this$filename.equals(other$filename)) return false;
final java.lang.Object this$dataId = this.getDataId();
final java.lang.Object other$dataId = other.getDataId();
if (this$dataId == null ? other$dataId != null : !this$dataId.equals(other$dataId)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Attachment;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + this.getClientId();
result = result * PRIME + this.getSize();
result = result * PRIME + this.getProjectId();
result = result * PRIME + this.getCreatedOn();
result = result * PRIME + this.getUserId();
result = result * PRIME + this.getLegacyId();
result = result * PRIME + (this.isImage() ? 79 : 97);
final java.lang.Object $filetype = this.getFiletype();
result = result * PRIME + ($filetype == null ? 43 : $filetype.hashCode());
final java.lang.Object $icon = this.getIcon();
result = result * PRIME + ($icon == null ? 43 : $icon.hashCode());
final java.lang.Object $entityId = this.getEntityId();
result = result * PRIME + ($entityId == null ? 43 : $entityId.hashCode());
final java.lang.Object $entityType = this.getEntityType();
result = result * PRIME + ($entityType == null ? 43 : $entityType.hashCode());
final java.lang.Object $filename = this.getFilename();
result = result * PRIME + ($filename == null ? 43 : $filename.hashCode());
final java.lang.Object $dataId = this.getDataId();
result = result * PRIME + ($dataId == null ? 43 : $dataId.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "Attachment(filetype=" + this.getFiletype() + ", icon=" + this.getIcon() + ", entityId=" + this.getEntityId() + ", clientId=" + this.getClientId() + ", entityType=" + this.getEntityType() + ", filename=" + this.getFilename() + ", size=" + this.getSize() + ", projectId=" + this.getProjectId() + ", createdOn=" + this.getCreatedOn() + ", dataId=" + this.getDataId() + ", userId=" + this.getUserId() + ", name=" + this.getName() + ", legacyId=" + this.getLegacyId() + ", isImage=" + this.isImage() + ", id=" + this.getId() + ")";
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Attachment(final String filetype, final String icon, final String entityId, final int clientId, final String entityType, final String filename, final int size, final int projectId, final int createdOn, final String dataId, final int userId, final String name, final int legacyId, final boolean isImage, final String id) {
this.filetype = filetype;
this.icon = icon;
this.entityId = entityId;
this.clientId = clientId;
this.entityType = entityType;
this.filename = filename;
this.size = size;
this.projectId = projectId;
this.createdOn = createdOn;
this.dataId = dataId;
this.userId = userId;
this.name = name;
this.legacyId = legacyId;
this.isImage = isImage;
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Attachment() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy