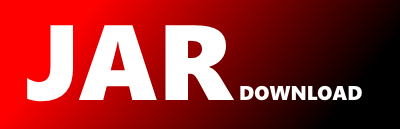
de.qytera.qtaf.testrail.entity.Attachments Maven / Gradle / Ivy
// Generated by delombok at Tue Jun 25 12:09:13 UTC 2024
package de.qytera.qtaf.testrail.entity;
import com.google.gson.annotations.SerializedName;
import java.util.List;
/**
* Multiple TestRail attachments.
*/
public class Attachments {
private List attachments;
private int offset;
private int size;
@SerializedName("_link")
private Link link;
private int limit;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAttachments() {
return this.attachments;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int getOffset() {
return this.offset;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int getSize() {
return this.size;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Link getLink() {
return this.link;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int getLimit() {
return this.limit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAttachments(final List attachments) {
this.attachments = attachments;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOffset(final int offset) {
this.offset = offset;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSize(final int size) {
this.size = size;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLink(final Link link) {
this.link = link;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLimit(final int limit) {
this.limit = limit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Attachments)) return false;
final Attachments other = (Attachments) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (this.getOffset() != other.getOffset()) return false;
if (this.getSize() != other.getSize()) return false;
if (this.getLimit() != other.getLimit()) return false;
final java.lang.Object this$attachments = this.getAttachments();
final java.lang.Object other$attachments = other.getAttachments();
if (this$attachments == null ? other$attachments != null : !this$attachments.equals(other$attachments)) return false;
final java.lang.Object this$link = this.getLink();
final java.lang.Object other$link = other.getLink();
if (this$link == null ? other$link != null : !this$link.equals(other$link)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Attachments;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + this.getOffset();
result = result * PRIME + this.getSize();
result = result * PRIME + this.getLimit();
final java.lang.Object $attachments = this.getAttachments();
result = result * PRIME + ($attachments == null ? 43 : $attachments.hashCode());
final java.lang.Object $link = this.getLink();
result = result * PRIME + ($link == null ? 43 : $link.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString() {
return "Attachments(attachments=" + this.getAttachments() + ", offset=" + this.getOffset() + ", size=" + this.getSize() + ", link=" + this.getLink() + ", limit=" + this.getLimit() + ")";
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Attachments(final List attachments, final int offset, final int size, final Link link, final int limit) {
this.attachments = attachments;
this.offset = offset;
this.size = size;
this.link = link;
this.limit = limit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Attachments() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy