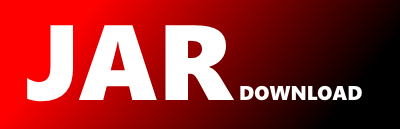
de.qytera.qtaf.testrail.utils.APIUtil Maven / Gradle / Ivy
// Generated by delombok at Tue Jun 25 12:09:13 UTC 2024
package de.qytera.qtaf.testrail.utils;
import de.qytera.qtaf.core.QtafFactory;
import jakarta.ws.rs.client.Entity;
import jakarta.ws.rs.core.MediaType;
import org.glassfish.jersey.media.multipart.FormDataContentDisposition;
import org.glassfish.jersey.media.multipart.FormDataMultiPart;
import org.glassfish.jersey.media.multipart.MultiPart;
import org.glassfish.jersey.media.multipart.file.FileDataBodyPart;
import java.io.File;
import java.io.IOException;
import java.text.ParseException;
import java.util.function.Consumer;
/**
* Utility class for handling TestRail multipart attachments.
*/
public class APIUtil {
/**
* Converts a file into a multipart attachment entity and passes the entity to the provided consumer.
*
* @param filePath the file to attach
* @param attachmentConsumer the consumer using the attachment entity
* @throws ParseException if the file cannot be processed
* @see TestRail documentation
*/
public static void consumeAttachment(String filePath, Consumer> attachmentConsumer) throws ParseException {
try (MultiPart multiPartEntity = new FormDataMultiPart()) {
FileDataBodyPart filePart = new FileDataBodyPart("attachment", new File(filePath));
// Testrail only accepts content-dispositions of the following form:
// Content-Disposition: form-data; name="attachment"; filename="..."
// See: https://github.com/gurock/testrail-api/blob/0320205d86f223415db3e8a301b9a534202ff820/java/com/gurock/testrail/APIClient.java#L166
filePart.setFormDataContentDisposition(new FormDataContentDisposition("form-data; name=\"attachment\"; filename=\"%s\"".formatted(filePart.getFileEntity().getName())));
multiPartEntity.bodyPart(filePart);
Entity entity = Entity.entity(multiPartEntity, MediaType.MULTIPART_FORM_DATA);
attachmentConsumer.accept(entity);
} catch (IOException e) {
QtafFactory.getLogger().error(e);
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
private APIUtil() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy