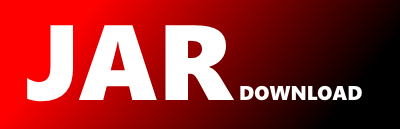
de.redsix.pdfcompare.InThreadExecutorService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdfcompare Show documentation
Show all versions of pdfcompare Show documentation
A simple Java library to compare two PDF files. Files are rendered and compared pixel by pixel.
package de.redsix.pdfcompare;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
public class InThreadExecutorService implements ExecutorService {
private boolean shutdown = false;
@Override
public void shutdown() {
shutdown = true;
}
@Override
public List shutdownNow() {
shutdown = true;
return Collections.emptyList();
}
@Override
public boolean isShutdown() {
return shutdown;
}
@Override
public boolean isTerminated() {
return shutdown;
}
@Override
public boolean awaitTermination(final long timeout, final TimeUnit unit) throws InterruptedException {
return true;
}
@Override
public Future submit(final Callable task) {
try {
return new ImmediateFuture<>(task.call());
} catch (Exception e) {
return new ImmediateFuture<>(e);
}
}
@Override
public Future submit(final Runnable task, final T result) {
try {
task.run();
} catch (Exception e) {
return new ImmediateFuture<>(e);
}
return new ImmediateFuture<>(result);
}
@Override
public Future> submit(final Runnable task) {
return submit(task, null);
}
@Override
public List> invokeAll(final Collection extends Callable> tasks) throws InterruptedException {
List> result = new ArrayList<>();
for (Callable task : tasks) {
result.add(submit(task));
}
return result;
}
@Override
public List> invokeAll(final Collection extends Callable> tasks, final long timeout, final TimeUnit unit)
throws InterruptedException {
return invokeAll(tasks);
}
@Override
public T invokeAny(final Collection extends Callable> tasks) throws InterruptedException, ExecutionException {
return null;
}
@Override
public T invokeAny(final Collection extends Callable> tasks, final long timeout, final TimeUnit unit)
throws InterruptedException, ExecutionException, TimeoutException {
return invokeAny(tasks);
}
@Override
public void execute(final Runnable command) {
command.run();
}
/*package for Testing*/ static class ImmediateFuture implements Future {
private final T result;
private final Exception exception;
public ImmediateFuture(final T result) {
this.result = result;
this.exception = null;
}
public ImmediateFuture(final Exception exeption) {
this.result = null;
this.exception = exeption;
}
@Override
public boolean cancel(final boolean mayInterruptIfRunning) {
return true;
}
@Override
public boolean isCancelled() {
return false;
}
@Override
public boolean isDone() {
return true;
}
@Override
public T get() throws InterruptedException, ExecutionException {
if (result != null) {
return result;
} else {
throw new ExecutionException(exception);
}
}
@Override
public T get(final long timeout, final TimeUnit unit) throws InterruptedException, ExecutionException, TimeoutException {
return get();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy