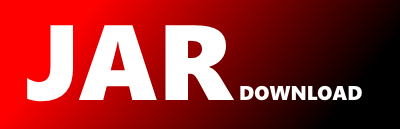
org.flexdock.docking.state.FloatManager Maven / Gradle / Ivy
The newest version!
/*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package org.flexdock.docking.state;
import java.awt.Component;
import java.awt.Rectangle;
import org.flexdock.docking.Dockable;
import org.flexdock.docking.floating.frames.DockingFrame;
/**
* This interface defines the API used for floating and grouping Dockables
. Classes
* implementing this interface will be responsible for sending Dockables
into
* DockingFrames
and managing the grouping of floating Dockables
.
*
* Sending a Dockable
into a floating DockingFrame
is relatively straightforward
* when supplied the Dockable
and a dialog owner. However, state must be maintained
* for each FloatingGroup
to allow the system to track which Dockables
* share the same floating dialog. If a floating Dockable
is closed and subsequently
* restored to its previous floating state, the FloatManager
must be able to determine
* whether an existing dialog is already present or a new dialog must be created into which the
* Dockable
may be restored. FloatingGroups
are used to track which
* dialogs contain which Dockables
. FloatManager
implementations must
* manage the addition to and removal of Dockables
from appropriate FloatingGroups
* and, in turn, use these FloatingGroups
to resolve or create the necessary
* DockingFrames
during float-operations.
*
* @author Christopher Butler
*/
public interface FloatManager {
FloatManager DEFAULT_STUB = new Stub();
FloatingGroup getGroup(String groupName);
FloatingGroup getGroup(Dockable dockable);
void addToGroup(Dockable dockable, String groupId);
void removeFromGroup(Dockable dockable);
DockingFrame floatDockable(Dockable dockable, Component frameOwner);
DockingFrame floatDockable(Dockable dockable, Component frameOwner, Rectangle screenBounds);
public static class Stub implements FloatManager {
@Override
public void addToGroup(Dockable dockable, String groupId) {
}
@Override
public DockingFrame floatDockable(Dockable dockable, Component frameOwner, Rectangle screenBounds) {
return null;
}
@Override
public DockingFrame floatDockable(Dockable dockable, Component frameOwner) {
return null;
}
@Override
public FloatingGroup getGroup(Dockable dockable) {
return null;
}
@Override
public FloatingGroup getGroup(String groupName) {
return null;
}
@Override
public void removeFromGroup(Dockable dockable) {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy