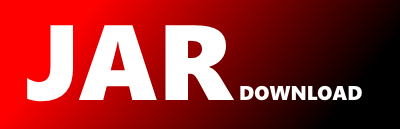
de.richtercloud.message.handler.DisplayUtils Maven / Gradle / Ivy
/**
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package de.richtercloud.message.handler;
import java.lang.reflect.InvocationTargetException;
import javafx.application.Platform;
import javax.swing.SwingUtilities;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
*
* @author richter
*/
public class DisplayUtils {
private final static Logger LOGGER = LoggerFactory.getLogger(DisplayUtils.class);
private final static Object JAVA_FX_RESULT_LOCK = new Object();
public static T displayOnJavaFXThread(DisplayCallable callable) {
if(Platform.isFxApplicationThread()) {
return callable.call();
}else {
EDTAnswer retValueHelper = new EDTAnswer<>();
//working with Conditions might be wrong here because both
//Condition.await and Condition.signal require the invoking thread
//to hold the lock the Condition is retrieved from which isn't
//possible here -> use wait and notify
Platform.runLater(() -> {
retValueHelper.setValue(callable.call());
synchronized(JAVA_FX_RESULT_LOCK) {
JAVA_FX_RESULT_LOCK.notifyAll();
}
});
try {
synchronized(JAVA_FX_RESULT_LOCK) {
JAVA_FX_RESULT_LOCK.wait();
}
} catch (InterruptedException ex) {
LOGGER.error("unexpected exception during processing of callable on JavaFX application thread",
ex);
throw new EDTExectutionException(ex);
}
return retValueHelper.getValue();
}
}
/**
* Invokes {@link DisplayCallable#call() } of {@code callable} if the
* invoking thread is the EDT or invokes {@code callable} on the EDT.
*
* @param the type of return value created by {@callable}
* @param callable the callable to produce the return value
* @return the return value created by the callable
*/
public static T displayOnEDT(DisplayCallable callable) {
if(SwingUtilities.isEventDispatchThread()) {
return callable.call();
}else {
EDTAnswer retValueHelper = new EDTAnswer<>();
try {
SwingUtilities.invokeAndWait(() -> {
retValueHelper.setValue(callable.call());
});
} catch (InterruptedException | InvocationTargetException ex) {
LOGGER.error("unexpected exception during processing of callable on EDT",
ex);
throw new EDTExectutionException(ex);
}
return retValueHelper.getValue();
}
}
private DisplayUtils() {
}
private static class EDTAnswer {
private T value;
protected EDTAnswer() {
}
public T getValue() {
return value;
}
public void setValue(T value) {
this.value = value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy