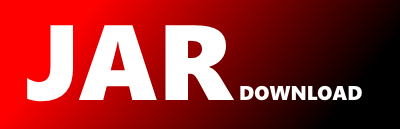
de.rpgframework.ConfigOption Maven / Gradle / Ivy
package de.rpgframework;
import java.util.Locale;
/**
* @author prelle
*
*/
public class ConfigOption {
public enum Type {
TEXT,
PASSWORD,
BOOLEAN,
NUMBER,
CHOICE,
MULTI_CHOICE,
/**
* A text containing a valid path to a directory.
*/
DIRECTORY,
/**
* A text containing a valid path to a file
*/
FILE,
}
private MultiLanguageResourceBundle RES;
private String id;
private Type type;
private T[] choiceOptions;
private T defaultValue;
private int lowerLimit;
private int upperLimit;
private StringConverter converter;
private transient T value;
//--------------------------------------------------------------------
public ConfigOption(String id, MultiLanguageResourceBundle i18n, Type type, T defVal) {
this.RES = i18n;
this.id = id;
this.type = type;
defaultValue = defVal;
}
//-------------------------------------------------------------------
public String getId() {
return id;
}
//-------------------------------------------------------------------
public String getName(Locale loc) {
String i18nKey = "config."+id;
return RES.getString(i18nKey, loc);
}
//--------------------------------------------------------------------
public T[] getChoiceOptions() {
return choiceOptions;
}
//-------------------------------------------------------------------
@SuppressWarnings("unchecked")
public ConfigOption setOptions(T... choices) {
choiceOptions = choices;
return this;
}
//-------------------------------------------------------------------
public String getOptionName(T obj, Locale loc) {
String i18nKey = "config."+id+".choice."+obj;
return RES.getString(i18nKey, loc);
}
//--------------------------------------------------------------------
public T getDefaultValue() {
return defaultValue;
}
//--------------------------------------------------------------------
public int getLowerLimit() {
return lowerLimit;
}
//--------------------------------------------------------------------
public Type getType() {
return type;
}
//--------------------------------------------------------------------
public int getUpperLimit() {
return upperLimit;
}
//-------------------------------------------------------------------
@SuppressWarnings({ "unchecked" })
public T getValue() {
if (value==null) return defaultValue;
return value;
// Preferences PREF = parent.getPreferences();
// switch (type) {
// case BOOLEAN:
// Boolean val = PREF.getBoolean(getLocalId(), (Boolean) defaultValue);
// return (T) val;
// case TEXT:
// case PASSWORD:
// T valChoice = (T) PREF.get(getLocalId(), null);
// if (valChoice==null)
// valChoice = defaultValue;
// return valChoice;
// case CHOICE:
// valChoice = null;
// String strChoice = PREF.get(getLocalId(), null);
// if (strChoice!=null && converter!=null)
// valChoice = converter.fromString(strChoice);
// if (valChoice==null && converter==null) {
// logger.warn("No converter for CHOICE string of "+getPathID()+" found");
// System.err.println("No converter for CHOICE string of "+getPathID()+" found");
// }
// if (valChoice==null)
// valChoice = defaultValue;
// return valChoice;
// case NUMBER:
// if (defaultValue instanceof Integer)
// return (T) (Integer)PREF.getInt(getLocalId(), (Integer) defaultValue);
// else if (defaultValue instanceof Float)
// return (T) (Float)PREF.getFloat(getLocalId(), (Float) defaultValue);
// else
// return (T) (Double)PREF.getDouble(getLocalId(), (Double) defaultValue);
// case MULTI_CHOICE:
// strChoice = PREF.get(getLocalId(), null);
// if (strChoice==null)
// return defaultValue;
// logger.debug("ConfigOptionImpl: convert to array: "+strChoice);
// StringTokenizer tok = new StringTokenizer(strChoice);
// List converted = new ArrayList();
// while (tok.hasMoreTokens()) {
// String token = tok.nextToken();
// if (converter!=null)
// converted.add(converter.fromString(token));
// else
// System.err.println("No converter for MULTICHOICE string of "+getPathID()+" found");
// }
// return (T) converted.toArray();
// default:
// return (T) PREF.get(getLocalId(), null);
// }
}
//-------------------------------------------------------------------
public String getStringValue() {
switch (type) {
case BOOLEAN:
return String.valueOf(getValue());
case TEXT:
case PASSWORD:
case CHOICE:
if (converter==null)
return (String)getValue();
return converter.toString(getValue());
case NUMBER:
return String.valueOf(getValue());
default:
return String.valueOf(getValue());
}
}
//-------------------------------------------------------------------
public void set(T newVal) {
//logger.info("set "+this.getName()+" to "+newVal);
this.value = newVal;
// Preferences PREF = parent.getPreferences();
// String oldVal = PREF.get(getLocalId(), null);
// if ((oldVal==null && newVal==null) || (oldVal!=null && newVal!=null && oldVal.equals(newVal)))
// return;
//
// if (newVal==null || String.valueOf(newVal).isEmpty())
// PREF.remove(getLocalId());
// else {
// switch (type) {
// case MULTI_CHOICE:
// List multiS = new ArrayList<>();
// if (newVal instanceof Object[]) {
// logger.debug("Array found");
// } else if (newVal instanceof List) {
// logger.debug("List found");
// for (T val : (List)newVal) {
// logger.debug(" list item "+val+" / "+val.getClass());
// if (converter!=null && !val.getClass().isEnum()) {
// multiS.add(converter.toString((T) val));
// } else
// multiS.add(String.valueOf(val));
// logger.debug(" multis now "+multiS);
// }
// newVal = String.join(" ", multiS);
// } else {
// logger.debug("No array");
// if (converter!=null)
// newVal = converter.toString((T) newVal);
// }
// PREF.put(getLocalId(), String.valueOf(newVal));
// break;
// default:
// PREF.put(getLocalId(), String.valueOf(newVal));
// }
// }
// logger.debug("Option "+getPathID()+" changed "+((type==Type.PASSWORD)?"":"to "+getValue())+" PREF KEY = "+getLocalId());
// super.parent.markRecentlyChanged(this);
// super.parent.fireConfigChange();
}
//-------------------------------------------------------------------
public ConfigOption setValueConverter(StringConverter converter) {
this.converter = converter;
return this;
}
//-------------------------------------------------------------------
public StringConverter getValueConverter() {
return converter;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy