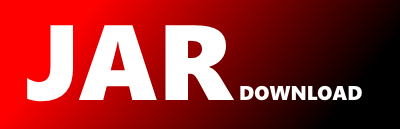
de.rpgframework.random.Actor Maven / Gradle / Ivy
The newest version!
package de.rpgframework.random;
import java.util.UUID;
import org.prelle.simplepersist.Attribute;
import org.prelle.simplepersist.Element;
import org.prelle.simplepersist.Root;
import de.rpgframework.classification.ActorRole;
import de.rpgframework.classification.Gender;
/**
* @author prelle
*
*/
@Root(name = "actor")
public class Actor extends VariableHolderNode {
@Attribute
private UUID id;
@Attribute
private ActorRole role;
@Element
private String name;
/** TODO: Gruppierung, zu der der Actor gehört */
private String faction;
@Element
private Gender gender;
@Element
private Object ruleData;
//-------------------------------------------------------------------
public Actor() {
id = UUID.randomUUID();
}
//-------------------------------------------------------------------
public Actor(ActorRole role, String name) {
id = UUID.randomUUID();
this.role = role;
this.name = name;
}
//-------------------------------------------------------------------
/**
* @return the name
*/
public String toString() {
return "Actor("+name+", var="+super.getGenericVariables()+")";
}
//-------------------------------------------------------------------
/**
* @return the name
*/
public String getName() {
return name;
}
//-------------------------------------------------------------------
/**
* @param name the name to set
*/
public void setName(String name) {
this.name = name;
}
//-------------------------------------------------------------------
/**
* @return the fraction
*/
public String getFaction() {
return faction;
}
//-------------------------------------------------------------------
/**
* @param fraction the fraction to set
*/
public void setFaction(String fraction) {
this.faction = fraction;
}
//-------------------------------------------------------------------
/**
* @return the id
*/
public UUID getId() {
return id;
}
//-------------------------------------------------------------------
/**
* @return the role
*/
public ActorRole getRole() {
return role;
}
//-------------------------------------------------------------------
/**
* @param role the role to set
*/
public void setRole(ActorRole role) {
this.role = role;
}
//-------------------------------------------------------------------
/**
* @return the gender
*/
public Gender getGender() {
return gender;
}
//-------------------------------------------------------------------
/**
* @param gender the gender to set
*/
public void setGender(Gender gender) {
this.gender = gender;
}
//-------------------------------------------------------------------
/**
* @return the ruleData
*/
public Object getRuleData() {
return ruleData;
}
//-------------------------------------------------------------------
/**
* @param ruleData the ruleData to set
*/
public void setRuleData(Object ruleData) {
this.ruleData = ruleData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy