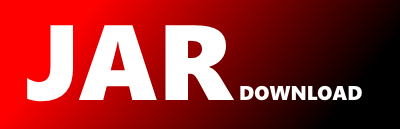
de.rpgframework.random.RollTable Maven / Gradle / Ivy
The newest version!
package de.rpgframework.random;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Random;
import java.util.StringTokenizer;
import org.prelle.simplepersist.Attribute;
import org.prelle.simplepersist.Element;
import org.prelle.simplepersist.ElementList;
import org.prelle.simplepersist.Root;
/**
* @author prelle
*/
@Root(name="rollTable")
public class RollTable {
/** An identifier within type */
@Attribute(required=true)
protected String id;
@Attribute
protected int d6;
@Attribute
protected int d20;
@Attribute
protected int d00;
@Attribute
protected String modifiers;
/** How many results shall be drawn from the table */
@Attribute
protected int draw = 1;
@Attribute
protected String nextTable;
@Element
private AddNodeInstruction addNode;
@ElementList(entry = "rollResult", type=RollResult.class, inline=true)
private List results;
//-------------------------------------------------------------------
public RollTable() {
results = new ArrayList<>();
}
//-------------------------------------------------------------------
public RollResult getResultFor(int value) {
List options = new ArrayList<>();
for (RollResult result : results) {
if (result.isInRange(value))
options.add(result);
}
// Select the result - roll again if multiple options
switch (options.size()) {
case 0: return null;
case 1: return options.get(0);
default:
return options.get( (new Random()).nextInt(options.size()));
}
}
//-------------------------------------------------------------------
/**
* @return the id
*/
public String getId() {
return id;
}
//-------------------------------------------------------------------
/**
* @return the d6
*/
public int getD6() {
return d6;
}
//-------------------------------------------------------------------
public Collection getModifierNames() {
List ret = new ArrayList<>();
if (modifiers!=null) {
StringTokenizer tok = new StringTokenizer(modifiers, ",");
while (tok.hasMoreTokens()) {
ret.add( tok.nextToken().trim() );
}
}
return ret;
}
//-------------------------------------------------------------------
/**
* @return the results
*/
public List getResults() {
return results;
}
//-------------------------------------------------------------------
/**
* @return the nextTable
*/
public String getNextTable() {
return nextTable;
}
//-------------------------------------------------------------------
/**
* @return the addNode
*/
public AddNodeInstruction getAddNode() {
return addNode;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy