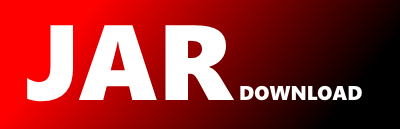
de.rpgframework.random.VariableHolderNode Maven / Gradle / Ivy
The newest version!
package de.rpgframework.random;
import java.lang.System.Logger;
import java.lang.System.Logger.Level;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.prelle.simplepersist.ElementList;
import de.rpgframework.classification.Classification;
import de.rpgframework.classification.ClassificationType;
import de.rpgframework.classification.DynamicClassification;
import de.rpgframework.core.RoleplayingSystem;
public class VariableHolderNode {
private final static Logger logger = System.getLogger(VariableHolderNode.class.getPackageName());
private RoleplayingSystem rules;
/**
* Defines general context information for a generator, like
* genre, type of location ...
*/
private Map> tags;
// @Element
private Map modifiers;
/**
* All values created by the generator that will be converted to text lines
*/
// @Element
private Map variables;
@ElementList(entry="line", type=TextLine.class)
private List lines;
//-------------------------------------------------------------------
public VariableHolderNode() {
modifiers = new HashMap<>();
variables = new HashMap();
lines = new ArrayList<>();
tags = new HashMap<>();
}
//-------------------------------------------------------------------
public VariableHolderNode(VariableHolderNode copy) {
modifiers = new HashMap<>();
variables = new HashMap();
copy.getGenericVariables().forEach( (k,v)-> variables.put(k, v));
lines = new ArrayList<>();
tags = new HashMap<>();
copy.getHints().forEach( c -> tags.put(c.getType(), c));
}
//-------------------------------------------------------------------
@SuppressWarnings("unchecked")
public T setHint(Classification> value) {
tags.put(value.getType(), value);
return (T)this;
}
//-------------------------------------------------------------------
@SuppressWarnings("unchecked")
public T setHint(ClassificationType type, String value) {
DynamicClassification dyn = new DynamicClassification(type, value);
tags.put(type, dyn);
return (T)this;
}
//-------------------------------------------------------------------
public VariableHolderNode withHints(List> data) {
data.forEach( c -> tags.put(c.getType(), c));
return this;
}
//-------------------------------------------------------------------
public List> getHints() {
return new ArrayList<>(tags.values());
}
//-------------------------------------------------------------------
public void copyHints(VariableHolderNode copy) {
copy.getHints().forEach( c -> tags.put(c.getType(), c));
}
//-------------------------------------------------------------------
public void copyVariables(VariableHolderNode copy) {
copy.getGenericVariables().forEach( (k,v)-> variables.put(k, v));
}
//-------------------------------------------------------------------
@SuppressWarnings("unchecked")
public T addVariable(GeneratorVariable modifier, int value) {
if (modifier==null)
throw new NullPointerException("modifier");
Integer old = modifiers.get(modifier);
if (old==null) {
modifiers.put(modifier, value);
} else {
modifiers.put(modifier, old+value);
}
return (T) this;
}
//-------------------------------------------------------------------
public VariableHolderNode withVariables(Map data) {
data.forEach( (k,v) -> modifiers.put(k, v));
return this;
}
//-------------------------------------------------------------------
public int getVariable(GeneratorVariable modifier) {
if (modifiers.containsKey(modifier))
return modifiers.get(modifier);
return 0;
}
//-------------------------------------------------------------------
public Map getVariables() {
return modifiers;
}
//-------------------------------------------------------------------
public Map getGenericVariables() {
return variables;
}
//-------------------------------------------------------------------
public VariableHolderNode setVariable(GeneratorVariable key, GeneratorVariableValue value) {
// if (key.isList()) {
// List prev = (List) variables.getOrDefault(key, new ArrayList());
// prev.add(value);
// variables.put(key, prev);
// } else {
variables.put(key, value);
// }
logger.log(Level.DEBUG, "Variable {0} of {1} now {2}", key, this, variables.get(key));
return this;
}
//-------------------------------------------------------------------
public VariableHolderNode setVariable(GeneratorVariable key, String value) {
StringGeneratorVariableValue var = new StringGeneratorVariableValue(null, key, value);
return setVariable(key, var);
}
//-------------------------------------------------------------------
public Object getGenericVariable(GeneratorVariable key) {
return variables.get(key);
}
//-------------------------------------------------------------------
/**
* @return the lines
*/
public List getLines() {
return lines;
}
//-------------------------------------------------------------------
/**
* @param lines the lines to set
*/
public void addLine(TextLine line) {
this.lines.add(line);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy