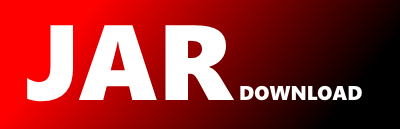
de.rpgframework.jfx.ChoiceManyDialog Maven / Gradle / Ivy
package de.rpgframework.jfx;
import java.lang.System.Logger;
import java.lang.System.Logger.Level;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
import java.util.Locale;
import org.prelle.javafx.CloseType;
import org.prelle.javafx.ManagedDialog;
import org.prelle.javafx.OptionalNodePane;
import org.prelle.javafx.SymbolIcon;
import org.prelle.javafx.WindowMode;
import de.rpgframework.genericrpg.chargen.PartialController;
import de.rpgframework.genericrpg.chargen.RecommendationState;
import de.rpgframework.genericrpg.chargen.RecommendingController;
import de.rpgframework.genericrpg.data.Choice;
import de.rpgframework.genericrpg.data.DataItem;
import de.rpgframework.genericrpg.data.DataItemValue;
import de.rpgframework.genericrpg.data.Decision;
import javafx.collections.ListChangeListener;
import javafx.scene.control.Label;
import javafx.scene.control.ListCell;
import javafx.scene.control.ListView;
import javafx.scene.control.SelectionMode;
import javafx.scene.layout.Priority;
import javafx.scene.layout.VBox;
/**
* Select one from a list of 2-3 choices
* @author prelle
*
*/
@SuppressWarnings("rawtypes")
public class ChoiceManyDialog extends ManagedDialog {
public final static Logger logger = System.getLogger(RPGFrameworkJFXConstants.BASE_LOGGER_NAME);
private String modText;
private DataItem decideFor;
private Choice choice;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy