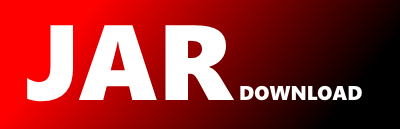
de.rpgframework.jfx.SelectingGridView Maven / Gradle / Ivy
package de.rpgframework.jfx;
import org.controlsfx.control.GridView;
import javafx.beans.property.ObjectProperty;
import javafx.beans.property.SimpleObjectProperty;
import javafx.collections.ObservableList;
import javafx.scene.control.MultipleSelectionModel;
import javafx.scene.control.SelectionMode;
/**
* @author prelle
*
*/
public class SelectingGridView extends GridView {
// --- Selection Model
private ObjectProperty> selectionModel = new SimpleObjectProperty>(this, "selectionModel");
//-------------------------------------------------------------------
/**
*/
public SelectingGridView() {
setSelectionModel(new StupidSimpleSingleSelectionModel<>(getItems()));
getSelectionModel().setSelectionMode(SelectionMode.SINGLE);
}
//-------------------------------------------------------------------
/**
* @param items
*/
public SelectingGridView(ObservableList items) {
super(items);
setSelectionModel(new StupidSimpleSingleSelectionModel<>(getItems()));
getSelectionModel().setSelectionMode(SelectionMode.SINGLE);
}
/**
* Sets the {@link MultipleSelectionModel} to be used in the ListView.
* Despite a ListView requiring a MultipleSelectionModel, it is possible
* to configure it to only allow single selection (see
* {@link MultipleSelectionModel#setSelectionMode(javafx.scene.control.SelectionMode)}
* for more information).
* @param value the MultipleSelectionModel to be used in this ListView
*/
public final void setSelectionModel(MultipleSelectionModel value) {
selectionModelProperty().set(value);
}
/**
* Returns the currently installed selection model.
* @return the currently installed selection model
*/
public final MultipleSelectionModel getSelectionModel() {
return selectionModel == null ? null : selectionModel.get();
}
/**
* The SelectionModel provides the API through which it is possible
* to select single or multiple items within a ListView, as well as inspect
* which items have been selected by the user. Note that it has a generic
* type that must match the type of the ListView itself.
* @return the selectionModel property
*/
public final ObjectProperty> selectionModelProperty() {
return selectionModel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy