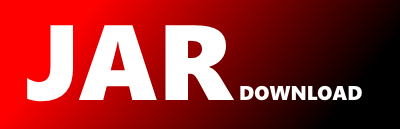
de.rpgframework.jfx.pages.CharacterExportPluginConfigPane Maven / Gradle / Ivy
package de.rpgframework.jfx.pages;
import java.io.File;
import java.lang.System.Logger;
import java.lang.System.Logger.Level;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Locale;
import java.util.ResourceBundle;
import org.prelle.javafx.FlexibleApplication;
import de.rpgframework.ConfigOption;
import de.rpgframework.ResourceI18N;
import de.rpgframework.genericrpg.export.CharacterExportPlugin;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.control.ChoiceBox;
import javafx.scene.control.Label;
import javafx.scene.control.PasswordField;
import javafx.scene.control.TextField;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.DirectoryChooser;
import javafx.util.StringConverter;
/**
* @author prelle
*
*/
public class CharacterExportPluginConfigPane extends VBox {
private final static Logger logger = System.getLogger(CharacterExportPluginSelectorPane.class.getPackageName());
public final static ResourceBundle RES = ResourceBundle.getBundle(CharacterViewLayout.class.getName());
private CharacterExportPlugin> plugin;
//-------------------------------------------------------------------
public CharacterExportPluginConfigPane(CharacterExportPlugin> plugin) {
this.plugin = plugin;
initComponents();
initLayout();
}
//-------------------------------------------------------------------
private void initComponents() {
}
//-------------------------------------------------------------------
private void initLayout() {
GridPane grid = new GridPane();
grid.setHgap(10);
grid.setVgap(10);
int i=0;
for (ConfigOption option : plugin.getConfiguration()) {
// DUmmy
if (option==null) {
logger.log(Level.ERROR,"STOP HERE - Option is NullPointer");
System.exit(1);
}
// // Ignore the config option that stores templates, since it is already being displayed
// if (option.getLocalId().equals("template"))
// continue;
i++;
grid.add(new Label(option.getName(Locale.getDefault())), 0, i);
switch (option.getType()) {
case TEXT:
TextField text = new TextField(String.valueOf(option.getValue()));
text.setId(option.getId());
text.setUserData(option);
text.textProperty().addListener( (ov,o,n) -> option.set(n));
grid.add(text, 1,i);
break;
case PASSWORD:
PasswordField pass = new PasswordField();
pass.setText(String.valueOf(option.getValue()));
pass.setId(option.getId());
pass.setUserData(option);
pass.textProperty().addListener( (ov,o,n) -> option.set(n));
grid.add(pass, 1,i);
break;
case CHOICE:
ChoiceBox
© 2015 - 2025 Weber Informatics LLC | Privacy Policy