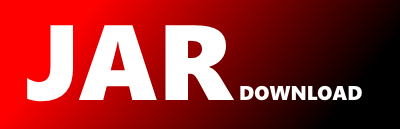
de.rpgframework.jfx.rules.AttributeTable Maven / Gradle / Ivy
package de.rpgframework.jfx.rules;
import java.util.function.BiFunction;
import de.rpgframework.character.RuleSpecificCharacterObject;
import de.rpgframework.genericrpg.NumericalValueController;
import de.rpgframework.genericrpg.data.AttributeValue;
import de.rpgframework.genericrpg.data.IAttribute;
import de.rpgframework.jfx.rules.skin.AttributeTableSkin;
import de.rpgframework.jfx.rules.skin.Properties;
import javafx.beans.property.ObjectProperty;
import javafx.beans.property.SimpleObjectProperty;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.fxml.FXML;
import javafx.scene.Node;
import javafx.scene.control.Control;
import javafx.scene.control.Skin;
/**
* @author Stefan Prelle
*
*/
public class AttributeTable extends Control {
public static class AttributeColumn {
private boolean beforeValueColumn;
private String title;
private BiFunction, A, Object> valueFactory;
private BiFunction componentFactory;
public AttributeColumn(BiFunction, A, Object> valueFact, BiFunction componentFact) {
beforeValueColumn = false;
this.valueFactory = valueFact;
this.componentFactory = componentFact;
}
public boolean isShowBeforeValueColumn() { return beforeValueColumn; }
public BiFunction, A, Object> getValueFactory() { return valueFactory; }
public BiFunction getComponentFactory() { return componentFactory; }
public void setTitle(String value) { this.title = value; }
public void setShowBeforeColumn(boolean value) { this.beforeValueColumn = true; }
public String getTitle() {return title;}
}
public enum Mode {
/**
* Show only the values, but don't allow any changes
*/
SHOW_ONLY,
/**
* Directly enter the attribute values
*/
DIRECT_INPUT,
/**
* Allow raising and lowering attributes with a Generator.
*/
GENERATE,
/**
* Allow raising and lowering attributes with a Controller
*/
CAREER,
}
@FXML
private ObjectProperty mode = new SimpleObjectProperty();
@FXML
private A[] attributes;
private ObjectProperty> model = new SimpleObjectProperty>();
private ObjectProperty selectedAttribute = new SimpleObjectProperty();
private ObjectProperty>> controller = new SimpleObjectProperty>>();
private ObservableList columns = FXCollections.observableArrayList();
//-------------------------------------------------------------------
public AttributeTable() {
}
//-------------------------------------------------------------------
public AttributeTable(A[] attributes) {
this.attributes = attributes;
this.mode.set(Mode.SHOW_ONLY);
}
//-------------------------------------------------------------------
public AttributeTable setAttributes(A[] attributes) {
return this;
}
//-------------------------------------------------------------------
/**
* @see javafx.scene.control.Control#createDefaultSkin()
*/
@Override
public Skin> createDefaultSkin() {
return new AttributeTableSkin(this);
}
//-------------------------------------------------------------------
public Mode getMode() { return mode.get(); }
public ObjectProperty modeProperty() { return mode; }
public AttributeTable setMode(Mode value) { mode.set(value); return this; }
public A[] getAttributes() { return attributes; }
//-------------------------------------------------------------------
public ObjectProperty> modelProperty() { return model; }
public RuleSpecificCharacterObject getModel() { return model.get(); }
public AttributeTable setModel(RuleSpecificCharacterObject value) { model.set(value); return this; }
//-------------------------------------------------------------------
public ObjectProperty selectedAttributeProperty() { return selectedAttribute; }
public IAttribute getSelectedAttribute() { return selectedAttribute.get(); }
public AttributeTable setSelectedAttribute(IAttribute value) { selectedAttribute.set(value); return this; }
//-------------------------------------------------------------------
public ObjectProperty>> controllerProperty() { return controller; }
public NumericalValueController> getController() { return controller.get(); }
public AttributeTable setController(NumericalValueController> value) { controller.set(value); return this; }
//-------------------------------------------------------------------
public ObservableList getColumns() { return columns; }
//-------------------------------------------------------------------
public void refresh() {
getProperties().put(Properties.RECREATE, Boolean.TRUE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy