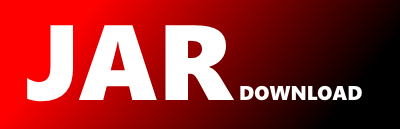
de.rpgframework.jfx.rules.skin.AttributeTableSkin Maven / Gradle / Ivy
package de.rpgframework.jfx.rules.skin;
import java.lang.System.Logger;
import java.util.HashMap;
import java.util.Map;
import org.prelle.javafx.JavaFXConstants;
import org.prelle.javafx.SymbolIcon;
import de.rpgframework.character.RuleSpecificCharacterObject;
import de.rpgframework.genericrpg.NumericalValueController;
import de.rpgframework.genericrpg.chargen.RecommendationState;
import de.rpgframework.genericrpg.data.AttributeValue;
import de.rpgframework.genericrpg.data.IAttribute;
import de.rpgframework.jfx.RPGFrameworkJFXConstants;
import de.rpgframework.jfx.rules.AttributeTable;
import de.rpgframework.jfx.rules.AttributeTable.AttributeColumn;
import de.rpgframework.jfx.rules.AttributeTable.Mode;
import javafx.collections.MapChangeListener;
import javafx.collections.ObservableMap;
import javafx.scene.Node;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.SkinBase;
import javafx.scene.layout.ColumnConstraints;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.Priority;
/**
* @author Stefan
*
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
public class AttributeTableSkin extends SkinBase> {
private final static Logger logger = System.getLogger(RPGFrameworkJFXConstants.BASE_LOGGER_NAME);
private class CellData {
public int columnNumber;
public Node lastComponent;
}
private class ColumnData {
public AttributeColumn colDef;
public Map cellData = new HashMap<>();
}
private GridPane grid;
private Map recIcon;
private Map finVal;
private Map decButton;
private Map incButton;
private Map customColumns;
private MapChangeListener
© 2015 - 2025 Weber Informatics LLC | Privacy Policy