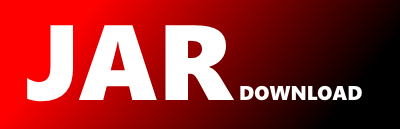
de.rpgframework.jfx.section.IconGridCell Maven / Gradle / Ivy
package de.rpgframework.jfx.section;
import java.util.function.Function;
import org.controlsfx.control.GridCell;
import de.rpgframework.genericrpg.data.DataItem;
import de.rpgframework.genericrpg.data.DataItemValue;
import de.rpgframework.jfx.RPGFrameworkJFXConstants;
import javafx.css.PseudoClass;
import javafx.scene.control.ContentDisplay;
import javafx.scene.control.SelectionModel;
import javafx.scene.control.Tooltip;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
public class IconGridCell> extends GridCell {
public static final PseudoClass PSEUDO_CLASS_SELECTED = PseudoClass.getPseudoClass("selected");
private final Image EMPTY = new Image(RPGFrameworkJFXConstants.class.getResourceAsStream("Person.png"));
private Function imageResolver;
private SelectionModel selectionModel;
private ImageView iView;
public IconGridCell(SelectionModel model, Function resolver) {
this.imageResolver = resolver;
this.selectionModel= model;
setContentDisplay(ContentDisplay.TOP);
setGraphicTextGap(0);
setStyle("-fx-text-fill: -fx-text-background-color; -fx-padding: 0 2px 0 2px; -fx-font-size:small");
iView = new ImageView();
iView.setFitHeight(IconSection.SIZE);
iView.setFitWidth(IconSection.SIZE);
initInteractivity();
}
//-------------------------------------------------------------------
private void initInteractivity() {
this.setOnMouseClicked(ev -> {
if (ev.getClickCount()==1) {
selectionModel.select(getItem());
this.pseudoClassStateChanged(PSEUDO_CLASS_SELECTED, true);
// if (oldState) {
// selectionModel.clearSelection(handles.indexOf(charac));
// } else {
// selectionModel.select(handles.indexOf(charac));
// }
}
});
}
//-------------------------------------------------------------------
/**
* @see javafx.scene.control.Cell#updateItem(java.lang.Object, boolean)
*/
@Override
public void updateItem(D item, boolean empty) {
super.updateItem(item, empty);
if (empty) {
setGraphic(null);
} else {
if (item != null) {
if (imageResolver != null) {
Image img = imageResolver.apply(item.getModifyable());
if (img != null) {
iView.setImage(img);
}
}
setText(item.getNameWithoutRating());
setTooltip(new Tooltip(item.getNameWithoutRating()));
} else {
iView.setImage(EMPTY);
}
setGraphic(iView);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy