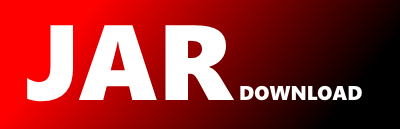
de.rpgframework.character.Attachment Maven / Gradle / Ivy
The newest version!
package de.rpgframework.character;
import java.nio.file.Path;
import java.util.Date;
import java.util.UUID;
public class Attachment {
public enum Format {
RULESPECIFIC,
RULESPECIFIC_EXTERNAL,
HTML,
TEXT,
PDF,
JSON,
BBCODE,
IMAGE
}
public enum Type {
CHARACTER,
BACKGROUND,
REPORT,
}
private transient CharacterHandle parent;
private UUID uuid;
private Type type;
private Format format;
private String name;
private transient byte[] data;
private Date modified;
private transient Object userData;
private transient Path localFile;
//-------------------------------------------------------------------
public Attachment(CharacterHandle parent, UUID uuid, Type type, Format format) {
this.parent = parent;
this.uuid = uuid;
this.type = type;
this.format = format;
}
//-------------------------------------------------------------------
public String toString() {
return uuid+"(name="+name+",type="+type+",format="+format+",data="+data+")";
}
//-------------------------------------------------------------------
public UUID getID() {
return uuid;
}
public Attachment setID(UUID value) { this.uuid = value; return this; }
//-------------------------------------------------------------------
public Type getType() { return type;}
public void setType(Type type) { this.type = type; }
//-------------------------------------------------------------------
public Format getFormat() { return format; }
public void setFormat(Format format) {this.format = format; }
//-------------------------------------------------------------------
public byte[] getData() { return data; }
//-------------------------------------------------------------------
/**
* Change the data within this attachment. Remember to call
* {@link de.rpgframework.character.CharacterProvider#modifyAttachment(CharacterHandle, Attachment)}
* to save the new data to the storage.
*
* @param data
* @see de.rpgframework.character.CharacterProvider#modifyAttachment(CharacterHandle, Attachment)
*/
public void setData(byte[] data) { this.data = data; }
//-------------------------------------------------------------------
public Date getLastModified() { return modified; }
public void setLastModified(Date date) { this.modified = date; }
//-------------------------------------------------------------------
public String getFilename() { return name; }
public void setFilename(String filename) { this.name = filename; }
//-------------------------------------------------------------------
/**
* @return the parsed
*/
public Object getParsed() { return userData; }
//-------------------------------------------------------------------
/**
* This may be used to store data of your liking - e.g. a {@link RuleSpecificCharacterObject}
* for the parsed data of a type=CHARACTER, format=RULESPECIFIC attachment.
* @param parsed Some user data
*/
public void setUserData(Object parsed) { this.userData = parsed; }
//-------------------------------------------------------------------
public CharacterHandle getParent() { return parent; }
public void setParent(CharacterHandle parent) { this.parent = parent;}
//-------------------------------------------------------------------
/**
* @return the localFile
*/
public Path getLocalFile() {
return localFile;
}
//-------------------------------------------------------------------
/**
* @param localFile the localFile to set
*/
public void setLocalFile(Path localFile) {
this.localFile = localFile;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy