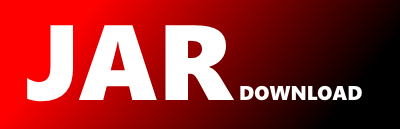
de.rpgframework.character.CharacterHandle Maven / Gradle / Ivy
/**
*
*/
package de.rpgframework.character;
import java.io.IOException;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.UUID;
import de.rpgframework.core.RoleplayingSystem;
/**
* @author prelle
*
*/
public abstract class CharacterHandle {
protected UUID uuid;
protected RoleplayingSystem rules;
protected String name;
protected String desc = "-";
protected Date lastModified;
protected boolean deleted;
protected boolean shared;
protected transient RuleSpecificCharacterObject,?,?,?> parsedCharac;
protected transient List attachments;
//-------------------------------------------------------------------
public CharacterHandle() {
attachments = new ArrayList<>();
}
//-------------------------------------------------------------------
public CharacterHandle(UUID uuid, RoleplayingSystem rules, String name, String desc, Date lastModified) {
super();
this.uuid = uuid;
this.rules = rules;
this.name = name;
this.desc = desc;
this.lastModified = lastModified;
attachments = new ArrayList<>();
}
//-------------------------------------------------------------------
public String getName() {
return name;
}
//-------------------------------------------------------------------
public String getShortDescription() {
if (parsedCharac!=null)
return parsedCharac.getShortDescription();
return desc;
}
//-------------------------------------------------------------------
public void setShortDescription(String desc) {
this.desc = desc;
}
//-------------------------------------------------------------------
public RoleplayingSystem getRuleIdentifier() {
return rules;
}
//-------------------------------------------------------------------
public Date getLastModified() {
return lastModified;
}
//-------------------------------------------------------------------
public UUID getUUID() {
return uuid;
}
public void setUUID(UUID value) {
this.uuid = value;
}
//-------------------------------------------------------------------
public void setName(String value) {
this.name= value;
}
//-------------------------------------------------------------------
public void setRuleIdentifier(RoleplayingSystem rules) {
this.rules = rules;
}
//-------------------------------------------------------------------
public void setLastModified(Date lastModified) {
this.lastModified = lastModified;
}
//--------------------------------------------------------------------
public RuleSpecificCharacterObject getCharacter() {
return parsedCharac;
}
//--------------------------------------------------------------------
public void setCharacter(RuleSpecificCharacterObject charac) throws IOException {
this.parsedCharac = charac;
}
//-------------------------------------------------------------------
public abstract Path getPath();
//-------------------------------------------------------------------
/**
* @return the deleted
*/
public boolean isDeleted() {
return deleted;
}
//-------------------------------------------------------------------
/**
* @param deleted the deleted to set
*/
public void setDeleted(boolean deleted) {
this.deleted = deleted;
}
//-------------------------------------------------------------------
/**
* @return the shared
*/
public boolean isShared() {
return shared;
}
//-------------------------------------------------------------------
/**
* @param shared the shared to set
*/
public void setShared(boolean shared) {
this.shared = shared;
}
//-------------------------------------------------------------------
/**
* Add a cached attachment
*/
public void addAttachment(Attachment att) {
if (att==null)
throw new NullPointerException();
attachments.add(att);
}
//-------------------------------------------------------------------
public void removeAttachment(Attachment att) {
attachments.remove(att);
}
//-------------------------------------------------------------------
public Collection getAttachments() {
return new ArrayList(attachments);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy