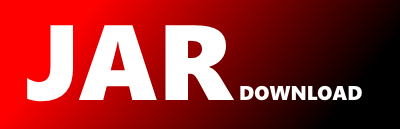
de.rpgframework.character.CharacterIOException Maven / Gradle / Ivy
The newest version!
package de.rpgframework.character;
import java.io.IOException;
/**
* @author prelle
*
*/
@SuppressWarnings("serial")
public class CharacterIOException extends IOException {
public enum ErrorCode {
FILESYSTEM_READ,
FILESYSTEM_WRITE,
ENCODING_FAILED,
DECODING_FAILED,
CHARACTER_WITH_THAT_NAME_EXISTS,
NO_WRITE_PERMISSION,
NO_FREE_ONLINE_CHARACTER_SLOTS,
MISSING_SERVER_CONNECTION,
OTHER_ERROR,
SERVER_ERROR
}
private ErrorCode code;
private String value;
private String path;
//-------------------------------------------------------------------
public CharacterIOException(ErrorCode code, String message) {
super(message);
this.code = code;
}
//-------------------------------------------------------------------
public CharacterIOException(ErrorCode code, String value, String message) {
super(message);
this.code = code;
this.value= value;
}
//-------------------------------------------------------------------
/**
* @param message
* @param cause
*/
public CharacterIOException(ErrorCode code, String message, Throwable cause) {
super(message, cause);
this.code = code;
}
//-------------------------------------------------------------------
/**
* @param message
* @param cause
*/
public CharacterIOException(ErrorCode code, String value, String message, Throwable cause) {
super(message, cause);
this.code = code;
this.value= value;
}
//-------------------------------------------------------------------
/**
* @param message
* @param cause
*/
public CharacterIOException(ErrorCode code, String value, String message, String path, Throwable cause) {
super(message, cause);
this.code = code;
this.value= value;
this.path = path;
}
//-------------------------------------------------------------------
/**
* @return the code
*/
public ErrorCode getCode() {
return code;
}
//-------------------------------------------------------------------
/**
* @return the value
*/
public String getValue() {
return value;
}
//-------------------------------------------------------------------
/**
* @return the path
*/
public String getPath() {
return path;
}
//-------------------------------------------------------------------
/**
* @param path the path to set
*/
public void setPath(String path) {
this.path = path;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy