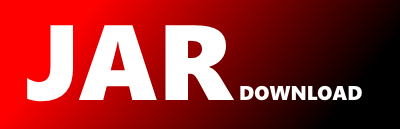
de.rpgframework.character.CharacterProvider Maven / Gradle / Ivy
The newest version!
/**
*
*/
package de.rpgframework.character;
import java.io.IOException;
import java.util.List;
import java.util.UUID;
import de.rpgframework.character.Attachment.Format;
import de.rpgframework.character.Attachment.Type;
import de.rpgframework.core.RoleplayingSystem;
/**
* This interface controls the storage of character related documents. The
* basic concept is that each character can have attachments of a specific
* {@link CharacterHandle.Format format} and {@link CharacterHandle.Type type}.
* Examples for those attachments may be portrait image (type CHARACTER, format IMAGE)
* serialized character data (type CHARACTER, format RULESPECIFIC) or a
* background story (type BACKGROUND, format PDF).
*
* All the attachments are collected in a {@link CharacterHandle}.
* CharacterHandles itself belong to a {@link de.rpgframework.core.Player} and
* have a {@link de.rpgframework.core.RoleplayingSystem} assigned.
*
*
* // Get the player that represents yourself
* Player myself = RPGFrameworkLoader.getInstance().getPlayerService().getMyself();
*
* // Obtain an instance of the character provider ...
* CharacterProvider charProv = RPGFrameworkLoader.getInstance().getCharacterProvider();
*
* // Obtain an instance of the character provider ...
* for (CharacterHandle handle : charProv.getCharacters(myself)) {
* // ... do something
* }
*
*
* @author prelle
*
*/
public interface CharacterProvider {
//-------------------------------------------------------------------
public void setListener(CharacterProviderListener callback);
//-------------------------------------------------------------------
/**
* Return an eventually existing character - or null if it doesn't exist yet.
* @param charName Name of the character
* @param ruleSystem The roleplaying system of the character
* @return The created handle
* @throws IOException Error executing operation - e.g. on directory creation or
* missing internet connection
*/
public CharacterHandle getCharacter(String charName, RoleplayingSystem ruleSystem) throws IOException;
//--------------------------------------------------------------------
/**
* Create a new CharacterHandle as a container for attachments for the
* local user.
*
* @param charName Name of the character
* @param ruleSystem The roleplaying system of the character
* @return The created handle
* @throws IOException Error executing operation - e.g. on directory creation or
* missing internet connection
*/
public CharacterHandle createCharacter(String charName, RoleplayingSystem ruleSystem) throws IOException;
//--------------------------------------------------------------------
/**
* Get a list of attachments without loading the data
* @param handle
* @return
*/
public List listAttachments(CharacterHandle handle) throws IOException;
//--------------------------------------------------------------------
/**
* Get a specific attachment with data
*/
public Attachment getFirstAttachment(CharacterHandle handle, Type type, Format format) throws IOException;
//--------------------------------------------------------------------
/**
* Adding an attachment to a character. Though the primary focus of this
* method is adding attachments only to characters of the local player,
* it may also be used for characters of other players as well. If this
* works is implementation dependant.
*
* How many instances (per format) of attachments are allowed, depends
* on the type: CHARACTER and BACKGROUND may only exist once, while
* REPORT may exist multiple times.
*
* @param handle Character to modify
* @param type What us described in this attachment
* @param format What kind of data is it
* @param filename A proposed file name. May be null. May be ignored
* by the implementation
* @param data Binary data of the attachment
* @return The created attachment
* @throws IOException Error executing operation
*/
public Attachment addAttachment(CharacterHandle handle, Type type, Format format, String filename, byte[] data) throws IOException;
// //--------------------------------------------------------------------
// /**
// * Copy the attachment from a different character provider to this one.
// * This method is used for synchronization.
// *
// * @param handle Character to modify
// * @param attach Attachment to copy
// * @return Cloned attachment
// * @throws IOException
// */
// public Attachment copyAttachment(CharacterHandle handle, Attachment attach) throws IOException;
//--------------------------------------------------------------------
/**
* Write modifications made to this attachment to storage.
*
* @param handle Character to modify
* @param attach Attachment that has been modified
* @throws IOException
*/
public void modifyAttachment(CharacterHandle handle, Attachment attach) throws IOException;
//--------------------------------------------------------------------
/**
* Remove an attachment from a character.
*
* @param handle Character to modify
* @param attach Attachment to remove
* @throws IOException
*/
public void deleteAttachment(CharacterHandle handle, Attachment attach) throws IOException;
//--------------------------------------------------------------------
/**
* Delete a character and all his attachments,
*
* @param handle Character to remove
* @throws IOException
*/
public void deleteCharacter(CharacterHandle handle) throws IOException;
//--------------------------------------------------------------------
/**
* Rename a character and all his attachments,
*
* @param handle Character to remove
* @throws IOException
*/
public void renameCharacter(CharacterHandle handle, String newName) throws IOException;
//--------------------------------------------------------------------
public List getMyCharacters() throws IOException;
//--------------------------------------------------------------------
/**
* Get all characters of a given player belonging to a specific roleplaying system.
*
* @param player Player to get characters from
* @param ruleSystem Roleplaying system to get characters for.
* @return A list of characters in no granted order.
*/
public List getMyCharacters(RoleplayingSystem ruleSystem) throws IOException;
//--------------------------------------------------------------------
public boolean isSynchronizeSupported();
//--------------------------------------------------------------------
public void initiateCharacterSynchronization();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy