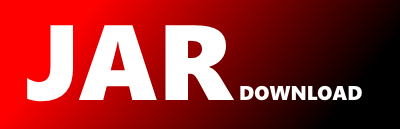
de.rpgframework.character.FileBasedCharacterHandle Maven / Gradle / Ivy
package de.rpgframework.character;
import java.io.IOException;
import java.lang.System.Logger;
import java.lang.System.Logger.Level;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Properties;
import java.util.UUID;
import java.util.function.Supplier;
import de.rpgframework.core.RoleplayingSystem;
/**
* @author prelle
*
*/
public class FileBasedCharacterHandle extends CharacterHandle {
private final static String NAME = "name";
final static String UUID = "uuid";
private final static String SYNC = "sync";
private final static String DESC = "desc";
protected transient static Logger logger = System.getLogger("eden.client");
private transient Path path;
private transient boolean sync = true;
private transient boolean loadAttemptMade = false;
//--------------------------------------------------------------------
public FileBasedCharacterHandle() {
}
//--------------------------------------------------------------------
public FileBasedCharacterHandle(Path path, RoleplayingSystem rules) {
super.uuid = java.util.UUID.randomUUID();
this.path = path;
super.rules = rules;
}
//--------------------------------------------------------------------
public FileBasedCharacterHandle(Path path, RoleplayingSystem rules, UUID uuid) {
super.uuid = uuid;
attachments = new ArrayList<>();
this.path = path;
super.rules = rules;
}
//--------------------------------------------------------------------
@Override
public String toString() {
return name+"@"+path+"/"+super.toString();
}
//--------------------------------------------------------------------
@Override
public boolean equals(Object o) {
if (o instanceof FileBasedCharacterHandle) {
FileBasedCharacterHandle other = (FileBasedCharacterHandle)o;
return toString().equals(other.toString());
}
return false;
}
//--------------------------------------------------------------------
/**
* @return the path
*/
public Path getPath() {
return path;
}
//-------------------------------------------------------------------
/**
* @param path the path to set
*/
public void setPath(Path path) {
if (path==null) {
throw new NullPointerException("Path must not be null");
}
this.path = path;
}
//-------------------------------------------------------------------
/**
* @return the sync
*/
public boolean hasSyncFlag() {
return sync;
}
//-------------------------------------------------------------------
/**
* @param sync the sync to set
*/
public void setSyncFlag(boolean sync) {
this.sync = sync;
}
//--------------------------------------------------------------------
public Properties getProperties() {
Properties pro = new Properties();
if (uuid!=null) {
pro.setProperty(UUID, uuid.toString());
}
pro.setProperty(SYNC, String.valueOf(sync));
pro.setProperty(DESC, getShortDescription());
pro.setProperty(NAME, getName());
// Convert attachments to property keys
for (Attachment attach : attachments) {
pro.setProperty("attachment."+attach.getID()+".type", attach.getType().name());
pro.setProperty("attachment."+attach.getID()+".format", attach.getFormat().name());
pro.setProperty("attachment."+attach.getID()+".file", attach.getFilename());
}
return pro;
}
//--------------------------------------------------------------------
public static Properties toProperties(CharacterHandle handle, Supplier> getAttachments) throws IOException {
Properties pro = new Properties();
if (handle.getUUID()!=null) {
pro.setProperty(UUID, handle.getUUID().toString());
}
List attachments = List.of();
if (handle instanceof FileBasedCharacterHandle) {
pro.setProperty(SYNC, String.valueOf(((FileBasedCharacterHandle)handle).hasSyncFlag()));
attachments = ((FileBasedCharacterHandle)handle).attachments;
} else {
attachments = getAttachments.get();
}
pro.setProperty(DESC, handle.getShortDescription());
pro.setProperty(NAME, handle.getName());
// Convert attachments to property keys
for (Attachment attach : attachments) {
pro.setProperty("attachment."+attach.getID()+".type", attach.getType().name());
pro.setProperty("attachment."+attach.getID()+".format", attach.getFormat().name());
pro.setProperty("attachment."+attach.getID()+".file", attach.getFilename());
}
return pro;
}
//--------------------------------------------------------------------
public static void fromProperties(CharacterHandle handle, Properties pro) {
logger.log(Level.DEBUG, "ENTER: fromProperties");
if (pro.containsKey(UUID))
handle.setUUID( java.util.UUID.fromString(pro.getProperty(UUID)) );
// if (pro.containsKey(SYNC))
// handle.setSyncFlag(Boolean.valueOf(pro.getProperty(SYNC, "false")));
if (pro.containsKey(DESC))
handle.setShortDescription(pro.getProperty(DESC));
if (pro.containsKey(NAME))
handle.setName(pro.getProperty(NAME));
if (handle instanceof FileBasedCharacterHandle) {
// Find out all filenames
List uuids = new ArrayList<>();
for (Object keyO : pro.keySet()) {
String key = (String)keyO;
if (key.startsWith("attachment.")) {
String file = key.substring(11, key.lastIndexOf("."));
if (!uuids.contains(file))
uuids.add(file);
}
}
// Now read all different Entries
FileBasedCharacterHandle fHandle = (FileBasedCharacterHandle)handle;
fHandle.attachments.clear();
for (String uuid : uuids) {
String typeS = pro.getProperty("attachment." + uuid + ".type");
String formatS = pro.getProperty("attachment." + uuid + ".format");
String filename = pro.getProperty("attachment." + uuid + ".file");
Path file = fHandle.path.resolve(filename);
if (Files.exists(file)) {
Attachment entry = new Attachment(handle, java.util.UUID.fromString(uuid), Attachment.Type.valueOf(typeS),
Attachment.Format.valueOf(formatS));
entry.setFilename(filename);
entry.setLocalFile(file);
try {
entry.setLastModified(new Date(Files.getLastModifiedTime(file).toMillis()));
} catch (IOException e) {
logger.log(Level.ERROR, "Failed getting modify time of " + file + ": " + e);
}
fHandle.attachments.add(entry);
} else {
logger.log(Level.WARNING, "Found metadata for non-existing file " + file + " in character " + handle.getName() + " - remove it");
pro.remove(typeS);
pro.remove(formatS);
pro.remove(uuid);
}
}
}
logger.log(Level.DEBUG, "ENTER: fromProperties");
}
//--------------------------------------------------------------------
public void setProperties(Properties pro) {
logger.log(Level.DEBUG, "ENTER: setProperties");
if (pro.containsKey(UUID))
uuid = java.util.UUID.fromString(pro.getProperty(UUID));
sync = Boolean.valueOf(pro.getProperty(SYNC, "false"));
desc = pro.getProperty(DESC, "-");
name = pro.getProperty(NAME);
// Find out all filenames
List uuids = new ArrayList<>();
for (Object keyO : pro.keySet()) {
String key = (String)keyO;
if (key.startsWith("attachment.")) {
String file = key.substring(11, key.lastIndexOf("."));
if (!uuids.contains(file))
uuids.add(file);
}
}
// Now read all different Entries
attachments.clear();
for (String uuid : uuids) {
String typeS = pro.getProperty("attachment." + uuid + ".type");
String formatS = pro.getProperty("attachment." + uuid + ".format");
String filename = pro.getProperty("attachment." + uuid + ".file");
if ("null".equals(uuid)) {
logger.log(Level.WARNING, "Found metadata with missing attachment UUID in character " + name + " - remove it");
pro.remove(typeS);
pro.remove(formatS);
pro.remove(uuid);
continue;
}
Path file = path.resolve(filename);
if (Files.exists(file)) {
Attachment entry = new Attachment(this, java.util.UUID.fromString(uuid), Attachment.Type.valueOf(typeS),
Attachment.Format.valueOf(formatS));
entry.setFilename(filename);
entry.setLocalFile(file);
try {
entry.setLastModified(new Date(Files.getLastModifiedTime(file).toMillis()));
} catch (IOException e) {
logger.log(Level.ERROR, "Failed getting modify time of " + file + ": " + e);
}
try {
entry.setData(Files.readAllBytes(file));
} catch (IOException e) {
logger.log(Level.ERROR, "Failed loading bytes from disk for "+file,e);
}
attachments.add(entry);
} else {
logger.log(Level.WARNING, "Found metadata for non-existing file " + file + " in character " + name + " - remove it");
pro.remove(typeS);
pro.remove(formatS);
pro.remove(uuid);
}
}
loadAttemptMade = true;
logger.log(Level.DEBUG, "ENTER: setProperties");
}
//-------------------------------------------------------------------
/**
* @return the loadAttemptMade
*/
public boolean isLoadAttemptMade() {
return loadAttemptMade;
}
//-------------------------------------------------------------------
/**
* @param loadAttemptMade the loadAttemptMade to set
*/
public void setLoadAttemptMade(boolean loadAttemptMade) {
this.loadAttemptMade = loadAttemptMade;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy