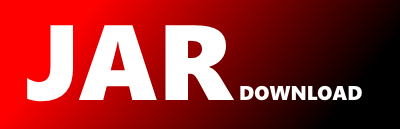
de.rpgframework.character.IUserDatabase Maven / Gradle / Ivy
The newest version!
package de.rpgframework.character;
import java.io.IOException;
import java.util.List;
import java.util.UUID;
/**
*
*/
public interface IUserDatabase {
//-------------------------------------------------------------------
public List getCharacters() throws IOException;
//-------------------------------------------------------------------
/**
* Modify a character in the database. If it doesn't exist, create it
*/
public void createCharacter(CharacterHandle handle) throws IOException;
public void modifyCharacter(CharacterHandle handle) throws IOException;
//-------------------------------------------------------------------
public void deleteCharacter(CharacterHandle handle) throws IOException;
//-------------------------------------------------------------------
/**
* Retrieve a character handle complete with all content-less attachments
* @param key Identifier of the character
* @return
*/
public CharacterHandle retrieveCharacter(UUID key) throws IOException;
//-------------------------------------------------------------------
public void createAttachment(CharacterHandle handle, Attachment attach) throws IOException;
//-------------------------------------------------------------------
public List getAttachments(CharacterHandle handle) throws IOException;
//-------------------------------------------------------------------
/**
* Modify a character in the database. If it doesn't exist, create it
*/
public void modifyAttachment(CharacterHandle handle, Attachment attach) throws IOException;
//-------------------------------------------------------------------
public void deleteAttachment(CharacterHandle handle, Attachment attach) throws IOException;
//-------------------------------------------------------------------
/**
* Retrieve data for an attachment
* @param key Identifier of the character
* @return
*/
public byte[] retrieveAttachment(CharacterHandle handle, Attachment attach) throws IOException;
public void modifyAttachmentData(CharacterHandle handle, Attachment attach) throws IOException;
public byte[] getAttachmentData(CharacterHandle handle, Attachment attach) throws IOException;
public List getDatasets() throws IOException;
public void storeDataset(DatasetDefinition value) throws IOException;
public void deleteDataset(DatasetDefinition value) throws IOException;
public byte[] getDatasetLocalization(DatasetDefinition value, String lang) throws IOException;
public void storeDatasetLocalization(DatasetDefinition value, String lang, byte[] data) throws IOException;
public void deleteDatasetLocalization(DatasetDefinition value, String lang) throws IOException;
public byte[] getDatasetFile(DatasetDefinition value, String name) throws IOException;
public void storeDatasetFile(DatasetDefinition value, String name, byte[] data) throws IOException;
public void deleteDatasetFile(DatasetDefinition value, String name) throws IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy