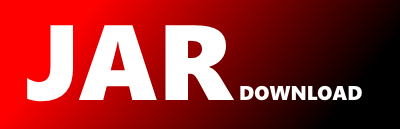
de.rpgframework.genericrpg.HistoryElement Maven / Gradle / Ivy
package de.rpgframework.genericrpg;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.Date;
import java.util.List;
import de.rpgframework.genericrpg.modification.DataItemModification;
public class HistoryElement {
/** Optional */
private String adventure;
private String name;
private List gained;
private List spent;
//-------------------------------------------------------------------
public HistoryElement() {
gained = new ArrayList();
spent = new ArrayList();
}
//-------------------------------------------------------------------
public String toString() {
return name+"(GAIN="+gained+", spent="+spent+")";
}
//-------------------------------------------------------------------
public void addGained(Reward mod) {
gained.add(mod);
Collections.sort(gained, new Comparator() {
public int compare(Reward o1, Reward o2) {
if (o1.getDate()!=null && o2.getDate()!=null)
return o1.getDate().compareTo(o2.getDate());
return 0;
}
});
}
//-------------------------------------------------------------------
public void addSpent(DataItemModification mod) {
spent.add(mod);
Collections.sort(spent, new Comparator() {
public int compare(DataItemModification o1, DataItemModification o2) {
if (o1.getDate()!=null && o2.getDate()!=null)
return o1.getDate().compareTo(o2.getDate());
return 0;
}
});
}
//-------------------------------------------------------------------
public Date getStart() {
if (gained.isEmpty())
return spent.get(0).getDate();
return gained.get(0).getDate();
}
//-------------------------------------------------------------------
public Date getEnd() {
if (gained.isEmpty())
return spent.get(spent.size()-1).getDate();
return gained.get(gained.size()-1).getDate();
}
//-------------------------------------------------------------------
public String getAdventureID() {
return adventure;
}
//-------------------------------------------------------------------
public String getName() {
return name;
}
//-------------------------------------------------------------------
/**
* @param name the name to set
*/
public void setName(String name) {
this.name = name;
}
//-------------------------------------------------------------------
public List getGained() {
return gained;
}
//-------------------------------------------------------------------
public List getSpent() {
return spent;
}
//-------------------------------------------------------------------
public int getTotalExperience() {
int sum = 0;
for (Reward reward : gained) sum+=reward.getExperiencePoints();
return sum;
}
//-------------------------------------------------------------------
public int getTotalMoney() {
int sum = 0;
for (Reward reward : gained) sum+=reward.getMoney();
return sum;
}
//-------------------------------------------------------------------
public List getGamemasters() {
List ret = new ArrayList<>();
for (Reward reward : gained) {
if (reward.getGamemaster()!=null && !ret.contains(reward.getGamemaster()))
ret.add(reward.getGamemaster());
}
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy