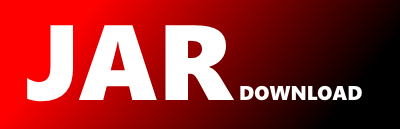
de.rpgframework.genericrpg.Possible Maven / Gradle / Ivy
The newest version!
package de.rpgframework.genericrpg;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.function.Function;
import de.rpgframework.MultiLanguageResourceBundle;
import de.rpgframework.genericrpg.ToDoElement.Severity;
import de.rpgframework.genericrpg.requirements.Requirement;
/**
* @author prelle
*
*/
public class Possible {
public enum State {
POSSIBLE(true),
DECISIONS_MISSING(true),
REQUIREMENTS_NOT_MET(false),
IMPOSSIBLE(false)
;
boolean value;
State(boolean val) {
value = val;
}
public boolean get() { return value; }
}
public static final Possible TRUE = new Possible(true);
public static final Possible FALSE = new Possible(false);
private State state;
private List req;
private List messages = new ArrayList<>();
//-------------------------------------------------------------------
public Possible(boolean value) {
this.state = value?State.POSSIBLE:State.IMPOSSIBLE;
req = new ArrayList<>();
}
//-------------------------------------------------------------------
public Possible(List unfulfilledReq, Function resolver) {
this.state = State.REQUIREMENTS_NOT_MET;
req = unfulfilledReq;
for (Requirement req : unfulfilledReq) {
messages.add(new ToDoElement(Severity.STOPPER, resolver.apply(req)));
}
}
//-------------------------------------------------------------------
public Possible(Requirement... unfulfilledReq) {
this.state = State.REQUIREMENTS_NOT_MET;
req = Arrays.asList(unfulfilledReq);
}
//-------------------------------------------------------------------
public Possible(Possible... all) {
state = State.POSSIBLE;
req = new ArrayList<>();
for (Possible tmp : all) {
if (tmp.getState()!=State.POSSIBLE) {
if (tmp.getState().ordinal()>state.ordinal())
state = tmp.state;
req.addAll(tmp.getUnfulfilledRequirements());
messages.addAll(tmp.getI18NKey());
}
}
}
//-------------------------------------------------------------------
public Possible(String i18n) {
this.state = State.IMPOSSIBLE;
this.messages.add(new ToDoElement(Severity.STOPPER, i18n));
req = new ArrayList<>();
}
//-------------------------------------------------------------------
public Possible(Severity severity, MultiLanguageResourceBundle res, String key, Object... params) {
this.state = (severity==Severity.STOPPER)?State.IMPOSSIBLE:State.DECISIONS_MISSING;
this.messages.add(new ToDoElement(severity, res, key, params));
req = new ArrayList<>();
}
//-------------------------------------------------------------------
public Possible(State state, Severity severity, MultiLanguageResourceBundle res, String key, Object... params) {
this.state = state;
this.messages.add(new ToDoElement(severity, res, key, params));
req = new ArrayList<>();
}
//-------------------------------------------------------------------
public Possible(boolean value, String i18n) {
this.state = value?State.POSSIBLE:State.IMPOSSIBLE;
req = new ArrayList<>();
// if (!value)
this.messages.add(new ToDoElement(Severity.STOPPER, i18n));
}
//-------------------------------------------------------------------
public Possible(State state, String i18n) {
this.state = state;
req = new ArrayList<>();
this.messages.add(new ToDoElement(Severity.STOPPER, i18n));
}
//-------------------------------------------------------------------
public List getUnfulfilledRequirements() {
return req;
}
//-------------------------------------------------------------------
public State getState() {
return state;
}
//-------------------------------------------------------------------
public boolean get() {
return state.get();
}
//-------------------------------------------------------------------
public boolean getRequireDecisions() {
if (state==State.DECISIONS_MISSING) return false;
return state.value;
}
//-------------------------------------------------------------------
public String toString() {
if (messages!=null && !messages.isEmpty())
return messages.get(0).getMessage();
return String.valueOf(state);
}
//-------------------------------------------------------------------
/**
* @return the message
*/
public List getI18NKey() {
return messages;
}
//-------------------------------------------------------------------
/**
* @return the message
*/
public ToDoElement getMostSevere() {
if (messages.isEmpty()) return null;
Collections.sort(messages);
return messages.get(0);
}
//-------------------------------------------------------------------
public ToDoElement getMostSevereExcept(List i18n) {
if (messages.isEmpty()) return null;
Collections.sort(messages);
for (ToDoElement mess : messages) {
if (mess.getKey()==null || !i18n.contains(mess.getKey()))
return mess;
}
return null;
}
//-------------------------------------------------------------------
/**
* @param state the state to set
*/
public void setState(State state) {
this.state = state;
}
//-------------------------------------------------------------------
public void addMessage(Severity severity, MultiLanguageResourceBundle res, String key, Object... params) {
this.messages.add(new ToDoElement(severity, res, key, params));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy