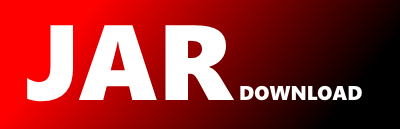
de.rpgframework.genericrpg.Reward Maven / Gradle / Ivy
The newest version!
package de.rpgframework.genericrpg;
import java.time.Instant;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.prelle.simplepersist.Attribute;
import org.prelle.simplepersist.Element;
import de.rpgframework.genericrpg.modification.DataItemModification;
import de.rpgframework.genericrpg.modification.Modification;
import de.rpgframework.genericrpg.modification.ModificationList;
import de.rpgframework.genericrpg.modification.ModifiedObjectType;
import de.rpgframework.genericrpg.modification.Modifyable;
public class Reward implements Datable, Modifyable {
@Attribute(name="exp")
private int experiencePoints;
@Attribute
private int money;
@Element
private String title;
@Element
private String gamemaster;
@Attribute(required=false)
protected Date date;
@Attribute(required=false)
private String id;
@Element
private ModificationList modifications;
//-------------------------------------------------------------------
public Reward() {
modifications = new ModificationList();
date = Date.from(Instant.now());
}
//-------------------------------------------------------------------
public String toString() {
return "Reward '"+title+"' at "+date+" (id="+id+")";
}
//-------------------------------------------------------------------
public String getTitle() {
return title;
}
//-------------------------------------------------------------------
public void setTitle(String title) {
this.title = title;
}
//-------------------------------------------------------------------
public List getIncomingModifications() {
return modifications;
}
//-------------------------------------------------------------------
public void setIncomingModifications(List mods) {
this.modifications = new ModificationList(mods);
}
//-------------------------------------------------------------------
public void addIncomingModification(Modification mod) {
// if (mod instanceof ModificationChoice) {
// if (((ModificationChoice)mod).getOptions().length>2 )
// throw new IllegalArgumentException("ModificationChoice without options: "+mod);
// }
modifications.add(mod);
}
//-------------------------------------------------------------------
public void removeIncomingModification(Modification mod) {
modifications.remove(mod);
}
//-------------------------------------------------------------------
public Date getDate() {
return date;
}
//-------------------------------------------------------------------
public void setDate(Date date) {
this.date = date;
}
//-------------------------------------------------------------------
public String getGamemaster() {
return gamemaster;
}
//-------------------------------------------------------------------
public void setGamemaster(String gamemaster) {
this.gamemaster = gamemaster;
}
//-------------------------------------------------------------------
/**
* @return the experiencePoints
*/
public int getExperiencePoints() {
return experiencePoints;
}
//-------------------------------------------------------------------
/**
* @param experiencePoints the experiencePoints to set
*/
public void setExperiencePoints(int experiencePoints) {
this.experiencePoints = experiencePoints;
}
//-------------------------------------------------------------------
/**
* @return the money
*/
public int getMoney() {
return money;
}
//-------------------------------------------------------------------
/**
* @param money the money to set
*/
public void setMoney(int money) {
this.money = money;
}
//-------------------------------------------------------------------
public void removeModification(ModifiedObjectType type, String ref) {
for (Modification tmp : new ArrayList<>(modifications)) {
if (tmp.getReferenceType()==type) {
DataItemModification mod = (DataItemModification)tmp;
if (mod.getKey().equals(ref)) {
modifications.remove(mod);
}
}
}
}
//-------------------------------------------------------------------
@SuppressWarnings("unchecked")
public D getModification(ModifiedObjectType type, String ref) {
for (Modification tmp : new ArrayList<>(modifications)) {
if (tmp.getReferenceType()==type) {
DataItemModification mod = (DataItemModification)tmp;
if (mod.getKey().equals(ref)) {
return (D) mod;
}
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy