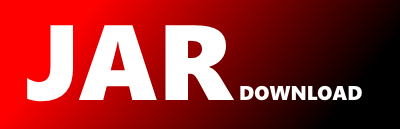
de.rpgframework.genericrpg.chargen.ComplexDataItemController Maven / Gradle / Ivy
package de.rpgframework.genericrpg.chargen;
import java.util.List;
import de.rpgframework.genericrpg.Possible;
import de.rpgframework.genericrpg.data.Choice;
import de.rpgframework.genericrpg.data.DataItem;
import de.rpgframework.genericrpg.data.DataItemValue;
import de.rpgframework.genericrpg.data.Decision;
/**
* @author prelle
*
*/
public interface ComplexDataItemController> extends PartialController {
public static enum RemoveMode {
// Treat as if it never has been selected (default for creation mode)
UNDO,
// Treat as if it was present, but has been removed now
REMOVE_LATE
}
//-------------------------------------------------------------------
/**
* Get all no yet selected items - either even with those whose
* requirements are not met, or without them-
* @param ignoreRequirements Also return items whose requirements are not met
* @return
*/
public List getAvailable();
//-------------------------------------------------------------------
/**
* Get a list of all currently selected items
*/
public List getSelected();
//-------------------------------------------------------------------
public RecommendationState getRecommendationState(D value);
public RecommendationState getRecommendationState(V value);
//-------------------------------------------------------------------
/**
* Get the choices to be made should the user want to select the item
* @param value Item to select
* @return List of choices to make
*/
public List getChoicesToDecide(D value);
//-------------------------------------------------------------------
/**
* Check if the user is allowed to select the item
* @param value Item to select
* @param decisions Decisions made
* @return Selection allowed or not
* @throws IllegalArgumentException Thrown if a decision is missing or invalid
*/
public Possible canBeSelected(D value, Decision... decisions);
//-------------------------------------------------------------------
/**
* Add/Select the item using the given decisions
* @param value Item to select
* @param decisions Decisions made
* @return value instance of selected item
* @throws IllegalArgumentException Thrown if a decision is missing or invalid
*/
public OperationResult select(D value, Decision... decisions);
//-------------------------------------------------------------------
/**
* Check if the user is allowed to deselect the item
* @param value ItemValue to deselect
* @return Deselection allowed or not
*/
public Possible canBeDeselected(V value);
//-------------------------------------------------------------------
/**
* Remove/Deselect the item (UNDO mode)
* @param value Item to select
* @return TRUE if item has been deselected
* @throws IllegalArgumentException Thrown if a decision is missing or invalid
*/
public boolean deselect(V value);
public default boolean deselect(V value, RemoveMode mode) {
return deselect(value);
}
//-------------------------------------------------------------------
public float getSelectionCost(D data, Decision... decisions);
//-------------------------------------------------------------------
default String getSelectionCostString(D data) {
float cost = getSelectionCost(data);
if (cost==0) return "";
return String.valueOf((int)cost);
}
// //-------------------------------------------------------------------
// public double getDeselectionCost(V value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy