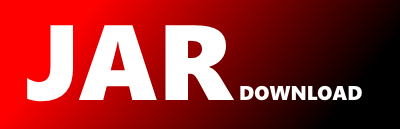
de.rpgframework.genericrpg.chargen.OperationResult Maven / Gradle / Ivy
package de.rpgframework.genericrpg.chargen;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import de.rpgframework.genericrpg.Possible;
import de.rpgframework.genericrpg.Possible.State;
import de.rpgframework.genericrpg.ToDoElement;
/**
* @author prelle
*
*/
public class OperationResult {
private List messages;
private T value;
//-------------------------------------------------------------------
public OperationResult() {
this.messages = new ArrayList<>();
messages.add(new ToDoElement(ToDoElement.Severity.STOPPER, "Not possible"));
}
//-------------------------------------------------------------------
public OperationResult(Possible poss) {
this.messages = new ArrayList<>();
if (poss.getState()==State.DECISIONS_MISSING) {
if (poss.getMostSevere()!=null)
messages.add(poss.getMostSevere());
}
if (poss.getState()==State.IMPOSSIBLE) {
if (poss.getI18NKey().isEmpty())
messages.add(new ToDoElement(ToDoElement.Severity.STOPPER, "Not possible"));
else
messages = poss.getI18NKey();
}
if (poss.getState()==State.REQUIREMENTS_NOT_MET)
messages.add(new ToDoElement(ToDoElement.Severity.WARNING, poss.getUnfulfilledRequirements()+""));
}
//-------------------------------------------------------------------
public OperationResult(Possible poss, boolean success) {
this.messages = new ArrayList<>();
if (!success) {
if (poss.getI18NKey().isEmpty())
messages.add(new ToDoElement(ToDoElement.Severity.STOPPER, "Not possible"));
else
messages = poss.getI18NKey();
}
if (poss.getState()==State.REQUIREMENTS_NOT_MET)
messages.add(new ToDoElement(ToDoElement.Severity.WARNING, poss.getUnfulfilledRequirements()+""));
}
//-------------------------------------------------------------------
public OperationResult(T value) {
this.messages = new ArrayList<>();
this.value = value;
}
//-------------------------------------------------------------------
public String toString() {
return String.valueOf(value);
}
//-------------------------------------------------------------------
public T get() {
return value;
}
//-------------------------------------------------------------------
public void set(T value) {
this.value = value;
}
//-------------------------------------------------------------------
public boolean hasError() {
return !messages.isEmpty();
}
//-------------------------------------------------------------------
public boolean wasSuccessful() {
return messages.isEmpty();
}
//-------------------------------------------------------------------
public List getMessages() {
return messages;
}
//-------------------------------------------------------------------
public String getError() {
return String.join("\n",messages.stream().map( m -> m.getMessage()).collect(Collectors.toList()));
}
//-------------------------------------------------------------------
public void addMessage(ToDoElement mess) {
messages.add(mess);
}
//-------------------------------------------------------------------
public boolean isPresent() {
return value!=null && messages.isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy