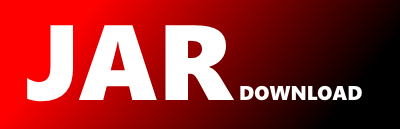
de.rpgframework.genericrpg.data.ASkillGroupValue Maven / Gradle / Ivy
The newest version!
package de.rpgframework.genericrpg.data;
import java.util.List;
import de.rpgframework.genericrpg.ModifyableNumericalValue;
import de.rpgframework.genericrpg.Pool;
import de.rpgframework.genericrpg.ValueType;
import de.rpgframework.genericrpg.modification.CheckModification;
import de.rpgframework.genericrpg.modification.Modification;
import de.rpgframework.genericrpg.modification.ValueModification;
/**
* @author Stefan
*
*/
public abstract class ASkillGroupValue extends DataItemValue implements ModifyableNumericalValue {
/**
* The final value of the attribute after generation, before
* exp have been spent.
* During priority generation this contains the value until
* which the attribute is paid by attribute points
*/
@org.prelle.simplepersist.Attribute(name="start",required=false)
protected int start;
// @ElementList(entry = "skillspec", type=SkillSpecializationValue.class, inline = true)
// protected List> specializations;
private transient Pool pool;
//-------------------------------------------------------------------
public ASkillGroupValue() {
// specializations = new ArrayList<>();
}
//-------------------------------------------------------------------
public ASkillGroupValue(S key) {
super(key);
// specializations = new ArrayList<>();
}
//-------------------------------------------------------------------
public ASkillGroupValue(S key, int distributed) {
super(key, distributed);
// specializations = new ArrayList<>();
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.ModifyableNumericalValue#getModifier(de.rpgframework.genericrpg.ValueType)
*/
@Override
public int getModifier(ValueType... typeArray) {
List types = List.of(typeArray);
int val = 0;
for (Modification mod : incomingModifications) {
if (mod instanceof CheckModification) {
continue;
}
if (mod instanceof ValueModification) {
ValueModification vMod = (ValueModification)mod;
if (types.contains( vMod.getSet() )) {
val += vMod.getValue();
}
}
}
return val;
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.ModifyableNumericalValue#getModifiedValue()
*/
@Override
public int getModifiedValue() {
return getModifiedValue(ValueType.NATURAL, ValueType.AUGMENTED);
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.ModifyableNumericalValue#getModifiedValue(de.rpgframework.genericrpg.ValueType)
*/
@Override
public int getModifiedValue(ValueType... typeArray) {
List types = List.of(typeArray);
boolean isNaturalOrAugmented = types.contains(ValueType.NATURAL) || types.contains(ValueType.AUGMENTED);
int val = isNaturalOrAugmented?value:0;
val += getModifier(typeArray);
return val;
}
//-------------------------------------------------------------------
/**
* @return the start
*/
public int getStart() {
return start;
}
//-------------------------------------------------------------------
/**
* @param start the start to set
*/
public void setStart(int start) {
this.start = start;
}
// //-------------------------------------------------------------------
// public List> getSpecializations() {
// return specializations;
// }
//
// public SkillSpecializationValue getSpecialization(SkillSpecialization spec) {
// for (SkillSpecializationValue tmp : specializations) {
// if (tmp.getModifyable()==spec)
// return tmp;
// }
// return null;
// }
//-------------------------------------------------------------------
/**
* @return the pool
*/
public Pool getPool() {
return pool;
}
//-------------------------------------------------------------------
/**
* @param pool the pool to set
*/
public void setPool(Pool pool) {
this.pool = pool;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy