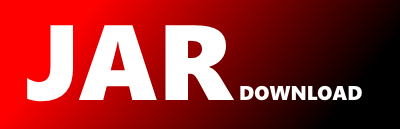
de.rpgframework.genericrpg.data.AttributeValue Maven / Gradle / Ivy
package de.rpgframework.genericrpg.data;
import de.rpgframework.genericrpg.ModifyableNumericalValue;
import de.rpgframework.genericrpg.Pool;
import de.rpgframework.genericrpg.ValueType;
import de.rpgframework.genericrpg.modification.Modification;
import de.rpgframework.genericrpg.modification.ModifyableImpl;
import de.rpgframework.genericrpg.modification.ValueModification;
/**
* @author prelle
*
*/
public class AttributeValue extends ModifyableImpl implements ModifyableNumericalValue {
@org.prelle.simplepersist.Attribute(name="id",required=true)
private A id;
/**
* The recent value of the attribute, including modifications by
* race on generation, the distributed points on generation
* and the points bought.
* Bought = unmodifiedValue - start;
*/
@org.prelle.simplepersist.Attribute(name="value",required=true)
private int distributed;
/**
* The final value of the attribute after generation, before
* exp have been spent.
* During priority generation this contains the value until
* which the attribute is paid by attribute points
*/
@org.prelle.simplepersist.Attribute(name="start",required=false)
private int start;
/**
* This is calculated by RPG implementations to follow their
* rules on limits and how things interoperate
*/
private transient Pool pool;
//-------------------------------------------------------------------
public AttributeValue() {
}
//-------------------------------------------------------------------
public AttributeValue(A key) {
id = key;
}
//-------------------------------------------------------------------
public AttributeValue(A key, int distributed) {
id = key;
this.distributed = distributed;
}
@Override
public String toString() {
return id+":"+distributed+"("+getIncomingModifications()+")";
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.NumericalValue#getDistributed()
*/
@Override
public int getDistributed() {
return distributed;
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.NumericalValue#setDistributed(int)
*/
@Override
public void setDistributed(int value) {
this.distributed = value;
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.SelectedValue#getModifyable()
*/
@Override
public A getModifyable() {
return id;
}
//-------------------------------------------------------------------
/**
* @return the start
*/
public int getStart() {
return start;
}
//-------------------------------------------------------------------
/**
* @param start the start to set
*/
public void setStart(int start) {
this.start = start;
}
//--------------------------------------------------------------------
public int getMaximum() {
int count = 0;
for (Modification mod : incomingModifications) {
if (mod instanceof ValueModification) {
ValueModification aMod = (ValueModification)mod;
if (aMod.getResolvedKey()==id && aMod.getSet()==ValueType.MAX)
count += aMod.getValue();
}
}
if (count<0) return 6+count;
return count;
// return Math.max(count, getNaturalModifier());
}
//--------------------------------------------------------------------
public String getDisplayString() {
int natural = getDistributed();
int augmented = getModifiedValue(ValueType.AUGMENTED);
int artificial= getModifiedValue(ValueType.ARTIFICIAL);
StringBuffer buf = new StringBuffer(String.valueOf(natural));
if (augmented!=natural)
buf.append("("+augmented+")");
if (artificial>0)
buf.append("|"+artificial);
return buf.toString();
// if (getModifier(ValueType.ARTIFICAL)>0)
// return getModifiedValue()+"("+getModifiedValue(ValueType.ARTIFICAL)+")";
// return String.valueOf(getModifiedValue());
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.ModifyableNumericalValue#getPool()
*/
public Pool getPool() {
return pool;
}
//-------------------------------------------------------------------
/**
* @param pool the pool to set
*/
public void setPool(Pool pool) {
this.pool = pool;
}
// //-------------------------------------------------------------------
// public void addModification(Modification mod) {
// if (mod.getSource()==null && mod.getWhen()!=ApplyWhen.ALLCREATE) throw new NullPointerException("No source");
// super.addModification(mod);
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy