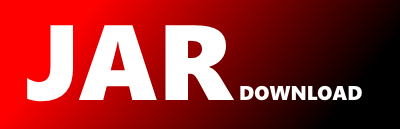
de.rpgframework.genericrpg.data.Choice Maven / Gradle / Ivy
The newest version!
package de.rpgframework.genericrpg.data;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.UUID;
import org.prelle.simplepersist.AttribConvert;
import org.prelle.simplepersist.Attribute;
import org.prelle.simplepersist.ElementList;
import de.rpgframework.genericrpg.items.Formula;
import de.rpgframework.genericrpg.items.formula.FormulaTool;
import de.rpgframework.genericrpg.modification.ModifiedObjectType;
import de.rpgframework.genericrpg.persist.DistributeConverter;
/**
* @author prelle
*
*/
public class Choice implements Cloneable {
/** What type of data is modified **/
@Attribute(required=true)
protected ModifiedObjectType type;
/** Optionally references a type from ModifiedObjectType */
@Attribute(required=false)
protected String ref;
/** Maximum value */
@Attribute(required=false)
protected String max;
private transient Formula parsedMax;
@Attribute(name="options")
protected String choiceOptions;
@Attribute(required=true)
protected UUID uuid;
@Attribute(name="neg", required = false)
private boolean negate;
/** CHOICE ONLY: How many choices can be made */
@Attribute
private int count;
/** CHOICE ONLY: The values to distribute (value-attribute is ignored) */
@Attribute(name="distr", required=false)
@AttribConvert(value = DistributeConverter.class)
private Integer[] distribute;
@Attribute(name="cost", required=false)
@AttribConvert(value = DistributeConverter.class)
private Integer[] costTable;
@ElementList(type = ChoiceOption.class, entry = "subOption", inline = true)
protected List subOptions = new ArrayList<>();
/** Use this key to get the name to use for this option */
@Attribute
private String i18n;
private transient ComplexDataItem source;
//-------------------------------------------------------------------
public Choice() {
}
//-------------------------------------------------------------------
public Choice(UUID uuid, ModifiedObjectType type) {
this.uuid = uuid;
this.type = type;
}
//-------------------------------------------------------------------
public Choice(UUID uuid, ModifiedObjectType type, String ref) {
this.uuid = uuid;
this.type = type;
this.ref = ref;
}
//-------------------------------------------------------------------
public Choice(String options) {
this.choiceOptions = options;
}
//-------------------------------------------------------------------
/**
* @see java.lang.Object#clone()
*/
@Override
public Object clone() {
Choice ret = new Choice();
ret.type = type;
ret.ref = ref;
ret.choiceOptions = choiceOptions;
ret.uuid = uuid;
ret.negate = negate;
ret.count = count;
ret.distribute = distribute;
ret.costTable = costTable;
ret.subOptions = new ArrayList<>(subOptions);
ret.source = source;
return ret;
}
//-------------------------------------------------------------------
public String toString() {
if (distribute!=null)
return uuid+"("+type+", ref="+ref+", options="+choiceOptions+", distr="+Arrays.toString(distribute)+")";
if (count>0)
return uuid+"("+type+", ref="+ref+", options="+choiceOptions+", count="+count+")";
return uuid+"("+type+", ref="+ref+", options="+choiceOptions+")";
}
//-------------------------------------------------------------------
/**
* @return the type
*/
public ModifiedObjectType getChooseFrom() {
return type;
}
//-------------------------------------------------------------------
/**
* @return the uuid
*/
public UUID getUUID() {
return uuid;
}
//-------------------------------------------------------------------
/**
* @return the uuid
*/
public String getTypeReference() {
return ref;
}
public void setTypeReference(String value) {
this.ref = value;
}
//--------------------------------------------------------------------
/**
* For choices
* @return The values to distribute (CHOICE only)
*/
public Integer[] getDistribute() { return distribute; }
//-------------------------------------------------------------------
public String getRawChoiceOptions() {
return choiceOptions;
}
//-------------------------------------------------------------------
public String[] getChoiceOptions() {
if (choiceOptions==null)
return null;
if (choiceOptions.contains(","))
return choiceOptions.trim().split(",");
return choiceOptions.trim().split(" ");
}
//-------------------------------------------------------------------
public void setChoiceOptions(String tokenizedString) {
choiceOptions = tokenizedString;
}
//-------------------------------------------------------------------
public void setChoiceOptions(String[] values) {
choiceOptions = String.join(",", values);
}
//-------------------------------------------------------------------
public boolean isNegated() {
return negate;
}
//-------------------------------------------------------------------
/**
* @return the count
*/
public int getCount() {
return count;
}
//-------------------------------------------------------------------
/**
* @return the source
*/
public ComplexDataItem getSource() {
return source;
}
//-------------------------------------------------------------------
/**
* @param source the source to set
*/
public void setSource(ComplexDataItem source) {
this.source = source;
}
//-------------------------------------------------------------------
/**
* @return the subOptions
*/
public List getSubOptions() {
return subOptions;
}
//-------------------------------------------------------------------
public ChoiceOption getSubOption(String subOption) {
if (subOptions!=null) {
for (ChoiceOption opt : subOptions) {
if (opt.getId().equals(subOption))
return opt;
}
}
return null;
}
//-------------------------------------------------------------------
public String getI18nKey() { return i18n; }
public void setI18NKey(String val) { this.i18n = val; }
//-------------------------------------------------------------------
public Formula getMaxFormula() {
if (parsedMax==null)
parsedMax = FormulaTool.tokenize(max);
return parsedMax;
}
//-------------------------------------------------------------------
public void setMaxFormula(Formula value) {
parsedMax = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy