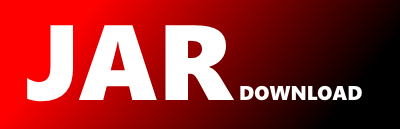
de.rpgframework.genericrpg.data.ChoiceOption Maven / Gradle / Ivy
package de.rpgframework.genericrpg.data;
import java.lang.System.Logger.Level;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Locale;
import java.util.MissingResourceException;
import org.prelle.simplepersist.Attribute;
import org.prelle.simplepersist.ElementList;
import de.rpgframework.genericrpg.items.ItemAttributeDefinition;
/**
* @author prelle
*
*/
@DataItemTypeKey(id="subOption")
public class ChoiceOption extends ComplexDataItem {
transient Choice choice;
transient ComplexDataItem parent;
@Attribute
private float cost;
@ElementList(entry = "attrdef", type = ItemAttributeDefinition.class, inline = true)
protected List attributes;
//-------------------------------------------------------------------
public ChoiceOption() {
attributes = new ArrayList<>();
}
//-------------------------------------------------------------------
/**
* @return the cost
*/
public float getCost() {
return cost;
}
//-------------------------------------------------------------------
/**
* @param cost the cost to set
*/
public void setCost(float cost) {
this.cost = cost;
}
//--------------------------------------------------------------------
@Override
public String getName(Locale locale) {
if (parent==null) return getLocalizedString(locale, "choice."+choice.ref+"."+id.toLowerCase());
String key = parent.getTypeString()+"."+parent.getId().toLowerCase()+".choice."+choice.ref+"."+id.toLowerCase();
return getLocalizedString(locale, key);
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.data.DataItem#getDescription(java.util.Locale)
*/
@Override
public String getDescription(Locale locale) {
String key = parent.getTypeString()+"."+parent.getId().toLowerCase()+".choice."+choice.ref+"."+id.toLowerCase()+".desc";
//String key = getTypeString()+"."+id.toLowerCase()+".desc";
if (parentSet==null) {
System.err.println("No parent dataset for "+getTypeString()+":"+id);
return key;
}
DataSet set = null;
int where = 0;
try {
set = getFirstParent(locale);
if (set==null)
return "?No ParentSet?";
where++;
String foo = set.getResourceString(key,locale);
return foo;
} catch (MissingResourceException mre) {
mre.printStackTrace();
if (where==0)
logger.log(Level.ERROR, mre.toString());
else
logger.log(Level.ERROR, "Missing resource "+mre.getKey()+"\t for locale "+locale+" in "+set.getBaseBundleName());
return id;
}
}
//-------------------------------------------------------------------
public Collection getAttributes() {
return attributes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy