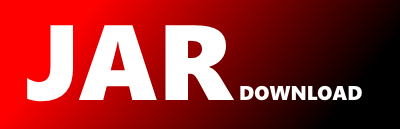
de.rpgframework.genericrpg.data.Decision Maven / Gradle / Ivy
The newest version!
package de.rpgframework.genericrpg.data;
import java.util.UUID;
import org.prelle.simplepersist.Attribute;
/**
* @author Stefan Prelle
*
*/
public class Decision {
@Attribute(name="choice", required=true)
private UUID choiceUUID;
@Attribute(required=true)
private String value;
private transient UUID valueAsUUID;
//-------------------------------------------------------------------
public Decision() {
}
//-------------------------------------------------------------------
public Decision(Choice choice, String value) {
if (choice.getUUID()==null)
throw new NullPointerException("Choice has no UUID");
this.choiceUUID = choice.getUUID();
this.value = value;
}
//-------------------------------------------------------------------
public Decision(UUID uuid, String value) {
this.choiceUUID = uuid;
this.value = value;
}
//-------------------------------------------------------------------
public Decision(String uuid, String value) {
this.choiceUUID = UUID.fromString(uuid);
this.value = value;
}
//-------------------------------------------------------------------
public Decision(UUID uuid, UUID value) {
if (uuid==null || value==null)
throw new NullPointerException();
this.choiceUUID = uuid;
this.value = value.toString();
}
//-------------------------------------------------------------------
/**
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return choiceUUID+"="+value;
}
//-------------------------------------------------------------------
/**
* @return the choiceUUID
*/
public UUID getChoiceUUID() {
return choiceUUID;
}
//-------------------------------------------------------------------
public String getValue() {
return value;
}
//-------------------------------------------------------------------
public int getValueAsInt() {
return Integer.parseInt(value);
}
//-------------------------------------------------------------------
/**
* @return the value
*/
public String[] getValues() {
return value.split(",");
}
//-------------------------------------------------------------------
public void setValue(String value) {
this.value = value;
}
//-------------------------------------------------------------------
/**
* @return the valueAsUUID
*/
public UUID getValueAsUUID() {
if (valueAsUUID==null) {
valueAsUUID = UUID.fromString(value);
}
return valueAsUUID;
}
//-------------------------------------------------------------------
/**
* @param valueAsUUID the valueAsUUID to set
*/
public void setValueAsUUID(UUID valueAsUUID) {
this.valueAsUUID = valueAsUUID;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy