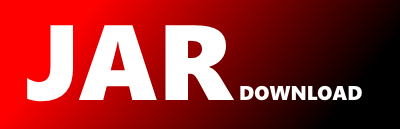
de.rpgframework.genericrpg.items.AAvailableSlot Maven / Gradle / Ivy
package de.rpgframework.genericrpg.items;
import java.util.ArrayList;
import java.util.List;
import org.prelle.simplepersist.Attribute;
import de.rpgframework.genericrpg.modification.Modification;
import de.rpgframework.genericrpg.modification.ModifyableImpl;
import de.rpgframework.genericrpg.modification.ValueModification;
/**
* @author prelle
*
*/
@SuppressWarnings("rawtypes")
public abstract class AAvailableSlot extends ModifyableImpl {
@Attribute
protected float capacity;
protected transient List> embedded;
//-------------------------------------------------------------------
protected AAvailableSlot() {
embedded = new ArrayList>();
}
//-------------------------------------------------------------------
public AAvailableSlot(float capacity) {
this();
this.capacity = capacity;
}
//-------------------------------------------------------------------
public List> getAllEmbeddedItems() {
return new ArrayList>(embedded);
}
//-------------------------------------------------------------------
public String toString() {
return getHook()+"(cap="+capacity+", used="+getUsedCapacity()+", items="+embedded+", mods="+incomingModifications+")";
}
//-------------------------------------------------------------------
/**
* @param accessory the accessory to set
*/
@SuppressWarnings("unchecked")
public void addEmbeddedItem(CarriedItem accessory) {
embedded.add(accessory);
}
//-------------------------------------------------------------------
public boolean removeEmbeddedItem(CarriedItem accessory) {
return embedded.remove(accessory);
}
//-------------------------------------------------------------------
/**
* Where is the slot attached
* @return the slot
*/
public abstract H getHook();
//-------------------------------------------------------------------
public float getCapacity() {
float modifier = 0;
for (Modification mod : getIncomingModifications()) {
if (mod instanceof ValueModification) {
modifier += ((ValueModification)mod).getFormula().getAsFloat();
}
}
return capacity + modifier;
}
//-------------------------------------------------------------------
public void setCapacity(float value) {
this.capacity = value;
}
//-------------------------------------------------------------------
public abstract float getUsedCapacity();
//-------------------------------------------------------------------
public abstract float getFreeCapacity();
//-------------------------------------------------------------------
public void clear() {
embedded.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy