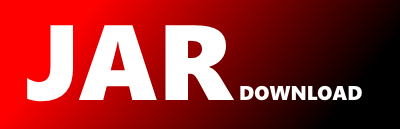
de.rpgframework.genericrpg.items.AGearData Maven / Gradle / Ivy
package de.rpgframework.genericrpg.items;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.prelle.simplepersist.ElementList;
import de.rpgframework.genericrpg.data.ComplexDataItem;
import de.rpgframework.genericrpg.data.DataItem;
import de.rpgframework.genericrpg.items.formula.FormulaImpl;
import de.rpgframework.genericrpg.items.formula.NumberElement;
import de.rpgframework.genericrpg.items.formula.ObjectElement;
/**
* @author prelle
*
*/
public abstract class AGearData extends ComplexDataItem implements Cloneable {
@ElementList(entry = "attrdef", type = ItemAttributeDefinition.class, inline = true)
protected List attributes;
/** Comma.separated list of flags */
@ElementList(entry = "flag", type = String.class, inline = false)
protected List flags;
/* Combination of direct and shortcut attributes */
protected transient Map cache;
// @Element
// protected Usage usage = new Usage();
/**
* Ways to apply this main/variant of an item.
* E.g. a Laser Sight might be mounted on top of a barrel, or under the
* barrel, but it is still the same item.
*/
@ElementList(entry = "usage", type = Usage.class, inline = true)
protected List usages;
//-------------------------------------------------------------------
public AGearData() {
cache = new HashMap<>();
attributes = new ArrayList<>();
usages = new ArrayList<>();
flags = new ArrayList<>();
userSelectableFlags = new ArrayList<>();
}
//-------------------------------------------------------------------
public abstract List extends IGearTypeData> getTypeData();
@SuppressWarnings("unchecked")
public T getTypeData(Class clazz) {
for (IGearTypeData tmp : getTypeData()) {
if (tmp.getClass()==clazz)
return (T) tmp;
}
return null;
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.data.DataItem#validate()
*/
@Override
public void validate() {
super.validate();
if (id==null)
throw new NullPointerException("id-Attribute not set for "+this);
for (IGearTypeData shortcut : getTypeData()) {
shortcut.copyToAttributes(this);
}
for (ItemAttributeDefinition tmp : attributes) {
cache.put(tmp.getModifyable(), tmp);
}
}
//-------------------------------------------------------------------
public Collection getAttributes() {
return cache.values();
}
//-------------------------------------------------------------------
public Collection getAttributes(String variantID) {
return cache.values();
}
//-------------------------------------------------------------------
public ItemAttributeDefinition getAttribute(IItemAttribute attrib) {
return cache.get(attrib);
}
//-------------------------------------------------------------------
public void setAttribute(ItemAttributeDefinition def) {
cache.put(def.getModifyable(), def);
}
//-------------------------------------------------------------------
public void setAttribute(IItemAttribute attrib, Formula value) {
if (value==null)
throw new NullPointerException("Cannnot set NULL for "+attrib);
ItemAttributeDefinition val = cache.get(attrib);
if (val==null) {
val = new ItemAttributeDefinition(attrib);
cache.put(attrib, val);
}
val.setRawValue(String.valueOf(value));
val.setFormula(value);
// if (value.getClass()==Integer.class || value.getClass()==int.class) {
// val.setValue( (int)value );
// } else {
// val.setValue(value);
// }
}
//-------------------------------------------------------------------
public void setAttribute(IItemAttribute attrib, int value) {
FormulaImpl form = new FormulaImpl();
form.addElement(new NumberElement(value, -1));
ItemAttributeDefinition val = cache.get(attrib);
if (val==null) {
val = new ItemAttributeDefinition(attrib);
cache.put(attrib, val);
}
val.setRawValue(String.valueOf(value));
val.setFormula(form);
}
//-------------------------------------------------------------------
public void setAttribute(IItemAttribute attrib, Object value) {
if (value instanceof Integer) {
setAttribute(attrib, (int)value);
return;
}
FormulaImpl form = new FormulaImpl();
form.addElement(new ObjectElement(value));
ItemAttributeDefinition val = cache.get(attrib);
if (val==null) {
val = new ItemAttributeDefinition(attrib);
cache.put(attrib, val);
}
if (value instanceof DataItem) {
val.setRawValue( ((DataItem)value).getId() );
} else {
val.setRawValue(String.valueOf(value));
}
val.setFormula(form);
}
//-------------------------------------------------------------------
public void addUsage(Usage usage) {
this.usages.add(usage);
}
//-------------------------------------------------------------------
/**
* @return the usage
*/
public List getUsages() {
// if (usages==null || usages.isEmpty())
// return List.of(new Usage());
return usages;
}
//-------------------------------------------------------------------
public Usage getUsage(CarryMode mode) {
for (Usage usage : getUsages()) {
if (usage.getMode()==mode)
return usage;
}
return null;
}
//-------------------------------------------------------------------
public List getFlags() {
return new ArrayList<>(flags);
}
//-------------------------------------------------------------------
public boolean hasFlag(String flag) {
return flags.contains(flag);
}
//-------------------------------------------------------------------
public void addFlags(List flags) {
// Sanity check
for (String flag : flags) {
if (flag==null || flag.isBlank())
throw new IllegalArgumentException("Null or empty flag in "+flags);
}
this.flags.addAll(flags);
}
// //-------------------------------------------------------------------
// /**
// * Return those attributes where the user needs to make a decision -
// * e.g. a rating
// */
// public List extends ItemAttributeDefinition> getAttributesToDecide() {
// return getAttributes().stream().filter(i -> (i.getMinimum()!=0 || i.getMaximum()!=0)).collect(Collectors.toList());
// }
//
// //-------------------------------------------------------------------
// /**
// * Return those attributes where the user needs to make a decision -
// * e.g. a rating
// */
// public List extends ItemAttributeDefinition> getAttributesToCalculate() {
// return getAttributes().stream().filter(i -> i.isFormula()).collect(Collectors.toList());
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy