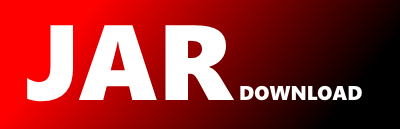
de.rpgframework.genericrpg.items.ItemAttributeDefinition Maven / Gradle / Ivy
package de.rpgframework.genericrpg.items;
import java.util.UUID;
import org.prelle.simplepersist.AttribConvert;
import org.prelle.simplepersist.Attribute;
import de.rpgframework.genericrpg.items.formula.FormulaImpl;
import de.rpgframework.genericrpg.items.formula.FormulaTool;
import de.rpgframework.genericrpg.modification.ModifyableImpl;
import de.rpgframework.genericrpg.persist.StringArrayConverter;
/**
* @author stefa
*
*/
public class ItemAttributeDefinition extends ModifyableImpl{
@org.prelle.simplepersist.Attribute(name="id",required=true)
private IItemAttribute id;
/**
* If value is the keyword 'CHOICE' expect the choice attribute to be present.
* If the lookupTable attribute is not NULL, expect the value to be
* an positive integer >0.
* */
@org.prelle.simplepersist.Attribute(name="value")
private String value;
private transient Formula parsedValue;
@Attribute(name="choice", required = false)
private UUID choice;
/** Lookup from parsed formula, before evaluating */
@org.prelle.simplepersist.Attribute(name="table")
@AttribConvert(value = StringArrayConverter.class)
private String[] lookupTable;
//-------------------------------------------------------------------
public ItemAttributeDefinition() {
// TODO Auto-generated constructor stub
}
//-------------------------------------------------------------------
public ItemAttributeDefinition(IItemAttribute key) {
id = key;
}
//-------------------------------------------------------------------
public String toString() {
return id+"="+value+"(parsed="+parsedValue+")";
}
//-------------------------------------------------------------------
public ItemAttributeDefinition(IItemAttribute key, Formula distributed) {
id = key;
this.parsedValue = distributed;
this.value = String.valueOf(distributed);
if (distributed.isFloat())
this.value = String.valueOf( distributed.getAsFloat() * 1000);
}
//-------------------------------------------------------------------
public IItemAttribute getModifyable() {
return id;
}
//-------------------------------------------------------------------
public Formula getFormula() {
if (parsedValue==null)
parsedValue = FormulaTool.tokenize(value);
return parsedValue;
}
//-------------------------------------------------------------------
public void setFormula(Formula value) {
parsedValue = value;
}
//-------------------------------------------------------------------
/**
* Returns the declaration like it appears in the XML definition.
* Use this method, if the value contains variables/formulas
*/
public String getRawValue() {
return value;
}
public void setRawValue(String val) {
value = val;
}
//-------------------------------------------------------------------
/** Shortcut for getFormula().getAsInteger()
*/
public int getDistributed() {
return getFormula().getAsInteger();
}
//-------------------------------------------------------------------
/** Shortcut for getFormula().getAsInteger()
*/
public T getValue() {
return getFormula().getAsObject(id.getConverter());
}
// //-------------------------------------------------------------------
// public boolean isFormula() {
// if (value==null)
// return false;
// return value.contains("$");
// }
//-------------------------------------------------------------------
public boolean isInteger() {
if (parsedValue==null) getFormula();
if (parsedValue!=null) {
return parsedValue.isInteger();
}
throw new IllegalStateException("Not resolved yet");
}
//-------------------------------------------------------------------
/**
* @return the lookupTable
*/
public String[] getLookupTable() {
return lookupTable;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy