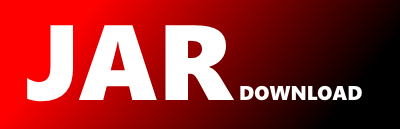
de.rpgframework.genericrpg.items.ItemAttributeFloatValue Maven / Gradle / Ivy
The newest version!
package de.rpgframework.genericrpg.items;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import java.util.Locale;
import java.util.stream.Collectors;
import de.rpgframework.genericrpg.ValueType;
import de.rpgframework.genericrpg.modification.ApplyableValueModification;
import de.rpgframework.genericrpg.modification.Modification;
import de.rpgframework.genericrpg.modification.ValueModification;
public class ItemAttributeFloatValue extends ItemAttributeValue {
@org.prelle.simplepersist.Attribute(name="value")
protected float value;
//-------------------------------------------------------------------
public ItemAttributeFloatValue(ItemAttributeDefinition template) {
super( (A)template.getModifyable());
if (!template.isInteger())
throw new IllegalArgumentException("Not a number definition");
this.value = template.getFormula().getAsFloat();
}
//-------------------------------------------------------------------
public ItemAttributeFloatValue(A attr) {
super(attr);
this.value = 0;
}
//-------------------------------------------------------------------
public ItemAttributeFloatValue(A attr, float val) {
super(attr);
this.value = val;
}
//-------------------------------------------------------------------
/**
* @see java.lang.Object#clone()
*/
@Override
public Object clone() {
ItemAttributeValue copy = new ItemAttributeFloatValue( this.attribute, this.value );
copy.addModifications( new ArrayList(this.incomingModifications) );
return copy;
}
//--------------------------------------------------------------------
public String toString() {
if (getModifier()==0)
return String.valueOf(value);
return value+" ("+getModifiedValue()+")";
}
//--------------------------------------------------------------------
// /**
// * @see de.rpgframework.genericrpg.ModifyableValue#getPoints()
// */
// @Override
public float getDistributed() {
return value;
}
//--------------------------------------------------------------------
// /**
// * @see de.rpgframework.genericrpg.ModifyableValue#setPoints(int)
// */
// @Override
public void setDistributed(float points) {
value = points;
}
//--------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.ModifyableValue#getModifier()
*/
public int getModifier() {
int count = 0;
for (Modification mod : incomingModifications) {
if (mod instanceof ApplyableValueModification) {
ApplyableValueModification sMod = (ApplyableValueModification)mod;
// if (sMod.getResolvedKey()==attribute)
// count += sMod.getValue();
} else if (mod instanceof ValueModification) {
count += ((ValueModification)mod).getValue();
}
}
return count;
}
//--------------------------------------------------------------------
public float getFloatModifier() {
BigDecimal count = new BigDecimal(0);
for (Modification mod : incomingModifications) {
if (mod instanceof ValueModification) {
ValueModification sMod = (ValueModification)mod;
BigDecimal d1 = new BigDecimal(String.format("%.3f", sMod.getValue()));
BigDecimal d2 = new BigDecimal("1000");
BigDecimal tmp = d1.divide(d2);
if (sMod.getResolvedKey()==attribute)
count = count.add(tmp);
}
}
return count.floatValue();
}
//--------------------------------------------------------------------
public BigDecimal getBigDecimalModifier() {
BigDecimal count = new BigDecimal(0);
for (Modification mod : incomingModifications) {
if (mod instanceof ValueModification) {
ValueModification sMod = (ValueModification)mod;
BigDecimal d1 = new BigDecimal(String.format("%d", sMod.getValue()));
BigDecimal d2 = new BigDecimal("1000");
BigDecimal tmp = d1.divide(d2);
if (sMod.getResolvedKey()==attribute)
count = count.add(tmp);
}
}
return count;
}
//--------------------------------------------------------------------
// /**
// * @see de.rpgframework.genericrpg.ModifyableValue#getModifiedValue()
// */
// @Override
public float getModifiedValue() {
BigDecimal b1 = new BigDecimal(String.format(Locale.ENGLISH, "%.3f", value));
// BigDecimal b1 = new BigDecimal(value);
BigDecimal b2 = getBigDecimalModifier();
return b1.add(b2).floatValue();
}
//--------------------------------------------------------------------
public double getModifiedValueDouble() {
BigDecimal b1 = new BigDecimal(String.format(Locale.ENGLISH, "%.3f", value));
BigDecimal b2 = getBigDecimalModifier();
return b1.add(b2).doubleValue();
}
//--------------------------------------------------------------------
public BigDecimal getModifiedValueBigDecimal() {
BigDecimal b1 = new BigDecimal(String.format(Locale.ENGLISH, "%.3f", value));
BigDecimal b2 = getBigDecimalModifier();
return b1.add(b2);
}
//-------------------------------------------------------------------
// /**
// * @see de.rpgframework.genericrpg.ModifyableNumericalValue#getModifiedValue(de.rpgframework.genericrpg.ValueType)
// */
// @Override
public float getModifiedValue(ValueType type) {
return getFloatModifier();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy