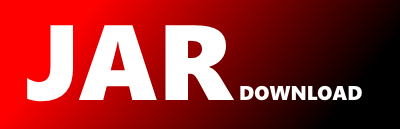
de.rpgframework.genericrpg.items.ItemAttributeNumericalValue Maven / Gradle / Ivy
package de.rpgframework.genericrpg.items;
import java.lang.System.Logger.Level;
import java.util.ArrayList;
import de.rpgframework.genericrpg.ModifyableNumericalValue;
import de.rpgframework.genericrpg.ValueType;
import de.rpgframework.genericrpg.modification.ApplyableValueModification;
import de.rpgframework.genericrpg.modification.Modification;
import de.rpgframework.genericrpg.modification.ValueModification;
public class ItemAttributeNumericalValue extends ItemAttributeValue implements
ModifyableNumericalValue {
@org.prelle.simplepersist.Attribute(name="value")
protected int value;
//-------------------------------------------------------------------
public ItemAttributeNumericalValue(ItemAttributeDefinition template) {
super( (A)template.getModifyable());
if (!template.isInteger())
throw new IllegalArgumentException("Not a number definition");
this.value = template.getFormula().getAsInteger();
}
//-------------------------------------------------------------------
public ItemAttributeNumericalValue(A attr) {
super(attr);
this.value = 0;
}
//-------------------------------------------------------------------
public ItemAttributeNumericalValue(A attr, int val) {
super(attr);
this.value = val;
}
//-------------------------------------------------------------------
/**
* @see java.lang.Object#clone()
*/
@Override
public Object clone() {
ItemAttributeNumericalValue copy = new ItemAttributeNumericalValue( this.attribute, value);
copy.addModifications( new ArrayList(this.incomingModifications) );
return copy;
}
//--------------------------------------------------------------------
public String toString() {
if (getModifier()==0)
return "NUM:"+String.valueOf(value);
return "NUM:"+value+" ("+getModifiedValue()+")";
}
//--------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.ModifyableValue#getPoints()
*/
@Override
public int getDistributed() {
return value;
}
//--------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.ModifyableValue#setPoints(int)
*/
@Override
public void setDistributed(int points) {
value = points;
}
//--------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.ModifyableValue#getModifier()
*/
public int getModifier() {
int count = 0;
for (Modification mod : incomingModifications) {
if (mod instanceof ApplyableValueModification) {
ApplyableValueModification sMod = (ApplyableValueModification)mod;
System.getLogger("de.rpgframework.genericrpg.items").log(Level.WARNING, "ToDo: ApplyableValueModification");
// if (sMod.getResolvedKey()==attribute)
// count += sMod.getValue();
} else if (mod instanceof ValueModification) {
ValueModification vMod = (ValueModification)mod;
if (vMod.getResolvedKey()==attribute) {
count += vMod.getValue();
} else {
System.getLogger("de.rpgframework.genericrpg.items").log(Level.WARNING, "mismatch of ItemAttribute");
}
} else {
System.getLogger("de.rpgframework.genericrpg.items").log(Level.WARNING, "Unsupported Modification: "+mod);
}
}
return count;
}
//--------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.ModifyableValue#getModifiedValue()
*/
@Override
public int getModifiedValue() {
return value + getModifier();
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.ModifyableNumericalValue#getModifiedValue(de.rpgframework.genericrpg.ValueType)
*/
@Override
public int getModifiedValue(ValueType... type) {
return getModifiedValue();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy