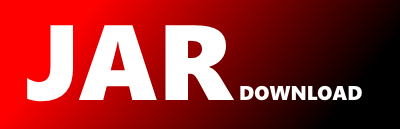
de.rpgframework.genericrpg.items.PieceOfGear Maven / Gradle / Ivy
The newest version!
package de.rpgframework.genericrpg.items;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.UUID;
import org.prelle.simplepersist.Attribute;
import org.prelle.simplepersist.ElementList;
import de.rpgframework.genericrpg.data.Choice;
import de.rpgframework.genericrpg.modification.ModifiedObjectType;
/**
* @author prelle
*
*/
public abstract class PieceOfGear,B extends AlternateUsage> extends AGearData {
public final static UUID VARIANT = UUID.randomUUID();
/**
* Other versions of this item you can buy
*/
@ElementList(entry = "variant", type = PieceOfGearVariant.class, inline = true)
protected List variants;
@Attribute(name="reqVariant")
protected boolean requireVariant;
/**
* Secondary ways to use this - like a dagger that can be thrown or used in close combat
*/
@ElementList(entry = "alternate", type = AlternateUsage.class, inline = true)
protected List alternates;
@Attribute
protected int price;
@ElementList(entry = "mode", type = OperationMode.class, inline = true)
protected List modes;
/**
* Can the gear only be picked by modifications (TRUE) or
* can the user freely select it (FALSE)
*/
@Attribute(name="modonly")
private boolean modOnly;
/**
* Is this an item where counting instances shall be possible?
*/
@Attribute(name="count")
protected boolean countable;
@Attribute
private Integer units;
private transient boolean validated;
protected transient ModifiedObjectType refType;
//-------------------------------------------------------------------
public PieceOfGear() {
variants = new ArrayList<>();
alternates = new ArrayList<>();
modes = new ArrayList<>();
}
//-------------------------------------------------------------------
public Collection getVariants() {
return variants;
}
//-------------------------------------------------------------------
public A getVariant(String id) {
for (A variant : variants) {
if (variant.getId().equals(id))
return variant;
}
return null;
}
//-------------------------------------------------------------------
public A getVariant(CarryMode carry) {
for (A variant : variants) {
if (variant.getUsage(carry)!=null)
return variant;
}
return null;
}
//-------------------------------------------------------------------
public void addVariant(A variant) {
if (!variants.contains(variant))
variants.add(variant);
}
//-------------------------------------------------------------------
@Override
public Collection getAttributes(String variantID) {
if (variantID==null)
return getAttributes();
LinkedHashMap temp = new LinkedHashMap<>();
cache.entrySet().forEach(entry -> temp.put(entry.getKey(), entry.getValue()));
// Now overwrite
PieceOfGearVariant variant = getVariant(variantID);
variant.cache.entrySet().forEach(entry -> temp.put(entry.getKey(), entry.getValue()));
return temp.values();
}
//-------------------------------------------------------------------
public Choice getChoice(UUID uuid) {
Choice ret = super.getChoice(uuid);
if (ret!=null)
return ret;
// Search in variants
for (A variant : variants) {
ret = variant.getChoice(uuid);
if (ret!=null) return ret;
}
return null;
}
//-------------------------------------------------------------------
public List getAlternates() {
return new ArrayList<>(alternates);
}
//-------------------------------------------------------------------
public List getOperationModes() {
return new ArrayList<>(modes);
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.data.DataItem#validate()
*/
@Override
public void validate() {
if (validated)
return;
super.validate();
for (PieceOfGearVariant variant : variants) {
variant.validate();
datasets.forEach(ds -> ds.getLocales().forEach(loc -> getVariantName(variant, loc)));
// getVariantName(variant, Locale.getDefault());
}
// Validate alternates
for (AlternateUsage> alt : alternates) {
alt.validate();
}
validated=true;
}
//-------------------------------------------------------------------
public String dump() {
StringBuffer buf = new StringBuffer(super.id);
for (ItemAttributeDefinition attr : super.cache.values()) {
buf.append("\n "+attr.getModifyable()+" : "+attr.isInteger()+" "+attr);
}
return buf.toString();
}
//-------------------------------------------------------------------
/**
* @return the modOnly
*/
public boolean isModOnly() {
return modOnly;
}
//-------------------------------------------------------------------
/**
* @return the countable
*/
public boolean isCountable() {
return countable;
}
//-------------------------------------------------------------------
public int getUnits() {
if (units==null) return 1;
return units;
}
//-------------------------------------------------------------------
public ModifiedObjectType getReferenceType() {
return refType;
}
//-------------------------------------------------------------------
public boolean requiresVariant() {
return requireVariant;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy