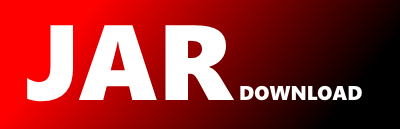
de.rpgframework.genericrpg.items.Usage Maven / Gradle / Ivy
The newest version!
package de.rpgframework.genericrpg.items;
import org.prelle.simplepersist.Attribute;
import de.rpgframework.genericrpg.items.formula.FormulaTool;
/**
* @author prelle
*
*/
public class Usage {
@Attribute
private CarryMode mode;
@Attribute
private Hook slot;
@Attribute
private String value;
/**
* Generic kind of additional info - may be interpreted by rulesystems
*/
@Attribute
private String type;
private transient Formula parsedValue;
//-------------------------------------------------------------------
public Usage() {
mode = CarryMode.CARRIED;
}
//-------------------------------------------------------------------
public Usage(CarryMode mode) {
this.mode = mode;
}
//-------------------------------------------------------------------
public Usage(CarryMode mode, Hook slot) {
this.mode = mode;
this.slot = slot;
}
//-------------------------------------------------------------------
public String toString() {
switch (mode) {
case CARRIED: return mode.name();
case EMBEDDED:
return mode.name()+" into "+slot+((value!=null)?(" for "+value+" capacity"):"");
case IMPLANTED:
return mode.name()+((value!=null)?(" for "+value+" essence"):"");
}
return mode.name();
}
//-------------------------------------------------------------------
/**
* @return the mode
*/
public CarryMode getMode() {
return mode;
}
//-------------------------------------------------------------------
/**
* @return the slot
*/
@SuppressWarnings("unchecked")
public H getSlot() {
return (H)slot;
}
//-------------------------------------------------------------------
public Formula getFormula() {
if (parsedValue==null && value!=null)
parsedValue = FormulaTool.tokenize(value);
return parsedValue;
}
//-------------------------------------------------------------------
public void setFormula(Formula value) {
parsedValue = value;
}
//-------------------------------------------------------------------
/**
* Returns the declaration like it appears in the XML definition.
* Use this method, if the value contains variables/formulas
*/
public String getRawValue() {
return value;
}
public void setRawValue(String val) {
value = val;
}
//-------------------------------------------------------------------
/** Shortcut for getFormula().getAsFloat()
*/
public float getSize() {
if (getFormula().isFloat())
return getFormula().getAsFloat();
return ((float)getFormula().getAsInteger())/1000;
}
//-------------------------------------------------------------------
/**
* Returns an uninterpreted type attribute
* @return the type
*/
public String getType() {
return type;
}
//-------------------------------------------------------------------
public void setSlot(H slot) {
this.slot = slot;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy