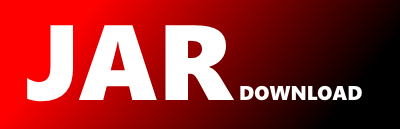
de.rpgframework.genericrpg.modification.DataItemModification Maven / Gradle / Ivy
The newest version!
package de.rpgframework.genericrpg.modification;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.UUID;
import org.prelle.simplepersist.Attribute;
import org.prelle.simplepersist.ElementList;
import org.prelle.simplepersist.Root;
import de.rpgframework.genericrpg.Datable;
import de.rpgframework.genericrpg.data.Decision;
import de.rpgframework.genericrpg.data.IReferenceResolver;
import de.rpgframework.genericrpg.data.ReferenceException;
/**
* A modification made to the current item it is attached to
* @author prelle
*
*/
@Root(name = "itemmod")
public class DataItemModification extends Modification implements Cloneable, Datable {
@Attribute(name="ref", required = true)
protected String ref;
@Attribute
protected String variant;
@Attribute(name="choice", required = false)
protected UUID choice;
@Attribute
protected boolean remove;
/**
* For modifications with a choice, this can limit the options
*/
@Attribute(name="options")
protected String choiceOptions;
/**
* Identifier of mode when the modification applies.
* Prepend a '!' to express a mode that may NOT be active
*/
@Attribute(name="mode", required=false)
protected String restrictedToMode;
@ElementList(type = Decision.class, entry = "decision", inline = true)
protected List decisions = new ArrayList<>();
@Attribute
protected int expCost;
@Attribute
protected Date date;
/**
* May be used to identify automatically added elements - e.g.
* like a lifestyle added to a character - and therefore prevent
* duplicate additions
*/
@Attribute
protected UUID id;
//-------------------------------------------------------------------
public DataItemModification() {
}
//-------------------------------------------------------------------
public DataItemModification(ModifiedObjectType type, String ref) {
this.type = type;
this.ref = ref;
}
//-------------------------------------------------------------------
public DataItemModification clone() {
return (DataItemModification) super.clone();
}
//-------------------------------------------------------------------
public boolean equals(Object o) {
if (o instanceof DataItemModification) {
DataItemModification other = (DataItemModification)o;
if (!super.equals(other)) return false;
if (ref!=null && !ref.equals(other.ref)) return false;
if (variant!=null && !variant.equals(other.variant)) return false;
return true;
}
return false;
}
//-------------------------------------------------------------------
/**
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return type+":"+ref+"(apply="+apply+",src="+source+")";
}
//-------------------------------------------------------------------
public String getKey() {
return ref;
}
//-------------------------------------------------------------------
public void setKey(String key) {
this.ref = key;
}
//-------------------------------------------------------------------
public T getResolvedKey() {
return type.resolve(ref);
}
//-------------------------------------------------------------------
public String[] getAsKeys() {
return ref.trim().split(",");
}
//-------------------------------------------------------------------
public UUID getConnectedChoice() {
return choice;
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.modification.Modification#validate()
*/
@Override
public void validate() throws ReferenceException {
if (ref.startsWith("CHOICE"))
return;
if ("ALL".equals(ref))
return;
if (type==null)
throw new RuntimeException("Modification has no type= attribute: "+this);
try {
Object val = getResolvedKey();
if (val==null && source instanceof IReferenceResolver) {
val = ((IReferenceResolver)source).resolveItem(ref);
}
if (val==null && ref.contains(",")) {
for (String k : getAsKeys()) {
val = type.resolve(k.trim());
if (val==null)
throw new ReferenceException(type, k);
}
}
if (val==null) {
if (ref.equals(ref.toUpperCase()))
return;
throw new ReferenceException(type, ref);
}
} catch (ReferenceException e) {
throw e;
}
// Validate decisions
for (Decision dec : decisions) {
UUID uuid = dec.getChoiceUUID();
if (uuid==null)
throw new RuntimeException("Decision without a choice UUID: "+this);
}
}
//-------------------------------------------------------------------
public List getDecisions() {
return decisions;
}
//-------------------------------------------------------------------
public Decision getDecision(UUID uuid) {
for (Decision dec : decisions) {
if (dec.getChoiceUUID().equals(uuid))
return dec;
}
return null;
}
//-------------------------------------------------------------------
public void setDecisions(List decisions) {
this.decisions = decisions;
}
//-------------------------------------------------------------------
/**
* @return the choiceOptions
*/
public String getChoiceOptions() {
return choiceOptions;
}
//-------------------------------------------------------------------
/**
* @return the expCost
*/
public int getExpCost() {
return expCost;
}
//-------------------------------------------------------------------
/**
* @param expCost the expCost to set
*/
public void setExpCost(int expCost) {
this.expCost = expCost;
}
//-------------------------------------------------------------------
/**
* @return the date
*/
public Date getDate() {
return date;
}
//-------------------------------------------------------------------
/**
* @param date the date to set
*/
public void setDate(Date date) {
this.date = date;
}
//-------------------------------------------------------------------
/**
* @return the remove
*/
public boolean isRemove() {
return remove;
}
//-------------------------------------------------------------------
/**
* @param remove the remove to set
*/
public void setRemove(boolean remove) {
this.remove = remove;
}
//-------------------------------------------------------------------
/**
* @return the variant
*/
public String getVariant() {
return variant;
}
//-------------------------------------------------------------------
/**
* @return the id
*/
public UUID getId() {
return id;
}
public void setId(UUID id) {
this.id =id;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy