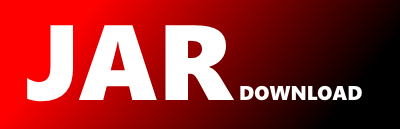
de.rpgframework.genericrpg.modification.EmbedModification Maven / Gradle / Ivy
package de.rpgframework.genericrpg.modification;
import java.util.UUID;
import org.prelle.simplepersist.Attribute;
import org.prelle.simplepersist.Root;
import de.rpgframework.genericrpg.data.ReferenceException;
import de.rpgframework.genericrpg.items.Hook;
/**
* Use this modification to put gear into available slots of other gear
* when defining gear in XML.
*
* @author prelle
*
*/
@Root(name="embed")
public class EmbedModification extends DataItemModification implements Cloneable{
/**
* This attribute contains the UUID of the gear
* to pick (since it often is variable, it cannot be directly put into
* the "choice" attribute.
*/
@Attribute
private UUID intoId;
@Attribute(name="intoType", required = true)
private ModifiedObjectType intoType;
/** Where to put item in - usually a HOOK */
@Attribute(name="intoRef", required = true)
private String intoRef;
@Attribute
private boolean included;
/** Shall this item be ignored for used capacity */
@Attribute(name="nocap")
private boolean ignoreForCapacity;
@Attribute(name="count")
private int count;
//-------------------------------------------------------------------
public EmbedModification() {
}
//-------------------------------------------------------------------
public T getHook() {
return intoType.resolve(intoRef);
}
//-------------------------------------------------------------------
/**
* Are effects of this item already included in the stats of the
* item is it added to
*/
public boolean isIncludedInStats() {
return included;
}
//-------------------------------------------------------------------
/**
* Shall the added item be ignored for used capacity calculation
*/
public boolean ignoreForCapacity() {
return ignoreForCapacity;
}
//-------------------------------------------------------------------
/**
* @see de.rpgframework.genericrpg.modification.Modification#validate()
*/
@Override
public void validate() throws ReferenceException {
super.validate();
try {
Hook val = getHook();
if (val==null)
throw new ReferenceException(type, ref);
} catch (ReferenceException e) {
throw e;
}
}
//-------------------------------------------------------------------
/**
* @return the count
*/
public int getCount() {
return count;
}
//-------------------------------------------------------------------
/**
* @param count the count to set
*/
public void setCount(int count) {
this.count = count;
}
//-------------------------------------------------------------------
/**
* @return the intoId
*/
public UUID getIntoId() {
return intoId;
}
//-------------------------------------------------------------------
/**
* @return the intoType
*/
public ModifiedObjectType getIntoType() {
return intoType;
}
//-------------------------------------------------------------------
/**
* @return the intoRef
*/
public String getIntoRef() {
return intoRef;
}
//-------------------------------------------------------------------
/**
* @return the included
*/
public boolean isIncluded() {
return included;
}
//-------------------------------------------------------------------
/**
* @return the ignoreForCapacity
*/
public boolean isIgnoreForCapacity() {
return ignoreForCapacity;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy