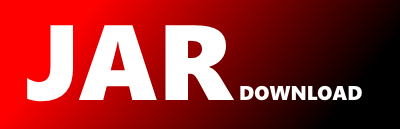
de.rpgframework.genericrpg.modification.Modification Maven / Gradle / Ivy
package de.rpgframework.genericrpg.modification;
import org.prelle.simplepersist.Attribute;
import de.rpgframework.genericrpg.data.ApplyTo;
import de.rpgframework.genericrpg.data.ApplyWhen;
import de.rpgframework.genericrpg.data.ReferenceException;
/**
* @author prelle
*
*/
public class Modification implements Cloneable {
public static enum Origin {
/** From child components */
CHILDREN,
/** From the character or parent components */
OUTSIDE,
}
/** What type of data is modified **/
@Attribute(required=false)
protected ModifiedObjectType type;
@Attribute(required=false)
protected ApplyTo apply;
@Attribute(required=false)
protected ApplyWhen when;
@Attribute(name="cond",required=false)
protected String conditions;
/**
* If the modification belongs to a module, this keeps the reference
*/
protected transient Object source;
protected transient Origin origin;
//-------------------------------------------------------------------
public Modification clone() {
try {
return (Modification) super.clone();
} catch ( CloneNotSupportedException e ) {
throw new InternalError();
}
}
//-------------------------------------------------------------------
public boolean equals(Object o) {
if (o instanceof Modification) {
Modification other = (Modification)o;
if (apply!=other.apply) return false;
if (conditions!=other.conditions) return false;
if (type!=other.type) return false;
if (when!=other.when) return false;
return true;
}
return false;
}
//-------------------------------------------------------------------
public Object getSource() { return source; }
public void setSource(Object src) { this.source = src; }
//-------------------------------------------------------------------
public boolean isConditional() {
return conditions!=null && !conditions.isBlank();
}
public void setConditionString(String value) { this.conditions = value; }
public String getConditionString() {
return conditions;
}
//-------------------------------------------------------------------
/**
* @return the type
*/
public ModifiedObjectType getReferenceType() {
return type;
}
//-------------------------------------------------------------------
/**
* @return the apply
*/
public ApplyTo getApplyTo() {
return apply;
}
//-------------------------------------------------------------------
/**
* @param when the when to set
*/
public void setApplyTo(ApplyTo apply) {
this.apply = apply;
}
//-------------------------------------------------------------------
/**
* @return the when
*/
public ApplyWhen getWhen() {
return when;
}
//-------------------------------------------------------------------
/**
* @param when the when to set
*/
public void setWhen(ApplyWhen when) {
this.when = when;
}
//-------------------------------------------------------------------
/**
* Validate content in this modification during load
*/
public void validate() throws ReferenceException {}
//-------------------------------------------------------------------
public Origin getOrigin() {
return origin;
}
//-------------------------------------------------------------------
public T setOrigin(Origin origin) {
this.origin = origin;
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy