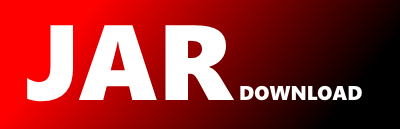
de.rpgframework.genericrpg.modification.ModificationChoice Maven / Gradle / Ivy
The newest version!
package de.rpgframework.genericrpg.modification;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
import java.util.UUID;
import org.prelle.simplepersist.Attribute;
import org.prelle.simplepersist.ElementList;
import org.prelle.simplepersist.ElementListUnion;
import org.prelle.simplepersist.Root;
import de.rpgframework.genericrpg.data.ReferenceException;
/**
* @author prelle
*
*/
@Root(name="selmod")
@ElementListUnion({
@ElementList(entry="allowmod", type=AllowModification.class),
@ElementList(entry="checkmod", type=CheckModification.class),
@ElementList(entry="costmod", type=CostModification.class),
@ElementList(entry="embed", type=EmbedModification.class),
@ElementList(entry="valmod", type=ValueModification.class),
@ElementList(entry="itemmod", type=DataItemModification.class),
@ElementList(entry="recommod", type=RecommendationModification.class),
@ElementList(entry="relevancemod", type=RelevanceModification.class),
@ElementList(entry="allmod", type=ModificationGroup.class),
})
public class ModificationChoice extends Modification implements List {
private List inner = new ArrayList<>();
@Attribute
private UUID uuid;
//-------------------------------------------------------------------
public ModificationChoice() {
}
//-------------------------------------------------------------------
public UUID getUUID() {
return uuid;
}
//-------------------------------------------------------------------
public DataItemModification getModification(UUID key) {
for (Modification tmp : inner) {
if ( tmp instanceof DataItemModification) {
DataItemModification mod = (DataItemModification)tmp;
if (key.equals(mod.getId()))
return mod;
}
}
return null;
}
public Iterator iterator() {
return inner.iterator();
}
//-------------------------------------------------------------------
public List getModificiations() {
return inner;
}
//-------------------------------------------------------------------
/**
* Validate all modifications in this list
*/
public void validate() throws ReferenceException {
inner.forEach(mod -> mod.validate());
}
@Override
public int size() {
return inner.size();
}
@Override
public boolean isEmpty() {
return inner.isEmpty();
}
@Override
public boolean contains(Object o) {
return inner.contains(o);
}
@Override
public Object[] toArray() {
return inner.toArray();
}
@Override
public T[] toArray(T[] a) {
return inner.toArray(a);
}
@Override
public boolean add(Modification e) {
return inner.add(e);
}
@Override
public boolean remove(Object o) {
return inner.remove(o);
}
@Override
public boolean containsAll(Collection> c) {
return inner.containsAll(c);
}
@Override
public boolean addAll(Collection extends Modification> c) {
return inner.addAll(c);
}
@Override
public boolean addAll(int index, Collection extends Modification> c) {
return inner.addAll(index, c);
}
@Override
public boolean removeAll(Collection> c) {
return inner.removeAll(c);
}
@Override
public boolean retainAll(Collection> c) {
return inner.retainAll(c);
}
@Override
public void clear() {
// TODO Auto-generated method stub
inner.clear();
}
@Override
public Modification get(int index) {
// TODO Auto-generated method stub
return inner.get(index);
}
@Override
public Modification set(int index, Modification element) {
// TODO Auto-generated method stub
return inner.set(index, element);
}
@Override
public void add(int index, Modification element) {
// TODO Auto-generated method stub
inner.add(index, element);
}
@Override
public Modification remove(int index) {
// TODO Auto-generated method stub
return inner.remove(index);
}
@Override
public int indexOf(Object o) {
// TODO Auto-generated method stub
return inner.indexOf(o);
}
@Override
public int lastIndexOf(Object o) {
// TODO Auto-generated method stub
return inner.lastIndexOf(o);
}
@Override
public ListIterator listIterator() {
// TODO Auto-generated method stub
return inner.listIterator();
}
@Override
public ListIterator listIterator(int index) {
// TODO Auto-generated method stub
return inner.listIterator(index);
}
@Override
public List subList(int fromIndex, int toIndex) {
// TODO Auto-generated method stub
return inner.subList(fromIndex, toIndex);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy